Rust Code Generation: A Complete Guide to Automated Development Tools and Macros - Related to demystifying, rust, complete, reflect, mastering
Demystifying Sorting Assertions With AssertJ
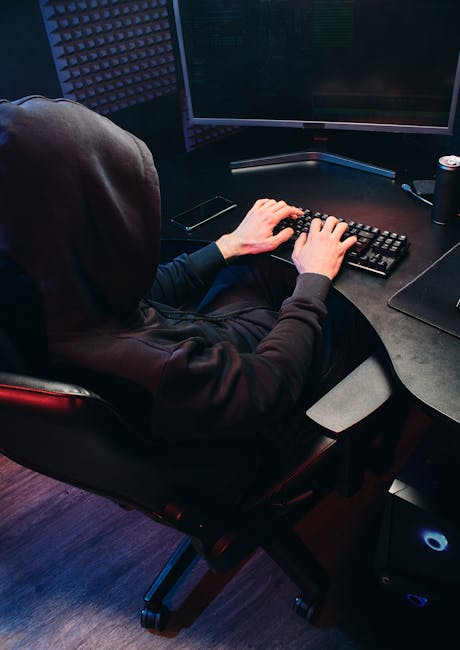
There are times when a new feature containing sorting is introduced. Obviously, we want to verify that the implemented sorting works correctly. AssertJ framework provides first-class support for such tasks. This article displays how to write such tests.
In this article, you will learn the following:
Two main methods provided by Assert frameworks for sorting assertion.
How to assert data sorted in ascending or descending way.
How to assert data sorted by multiple attributes.
How to deal with null s or case-insensitive sorting.
First of all, we need to know that AssertJ provides the AbstractListAssert abstract class for asserting any List argument. This class also contains the isSorted and isSortedAccordingTo methods for our purpose. The solution used there is based on the Comparator interface and its several static methods. Before moving to our examples, let's have a short introduction to the data and technology used in this article.
Almost every case presented here is demonstrated with two examples. The simple one is always presented first, and it's based on a list of String values. The goal is to provide the simplest example which can be easily taken and tested. However, the second example is based on the DB solution introduced in the Introduction: Querydsl vs. JPA Criteria article. There, we use Spring Data JPA solution for the PDM model defined as:
These tables are mapped to City and Country entities. Their implementation is not mentioned here, as it's available in the article mentioned earlier. Nevertheless, the data used in the examples below are defined like this:
Plain Text [ City(id=5, name=Atlanta, state=Georgia, country=Country(id=3, name=USA)), City(id=13, name=Barcelona, state=Catalunya, country=Country(id=7, name=Spain)), City(id=14, name=Bern, state=null, country=Country(id=8, name=Switzerland)), City(id=1, name=Brisbane, state=Queensland, country=Country(id=1, name=Australia)), City(id=6, name=Chicago, state=Illionis, country=Country(id=3, name=USA)), City(id=15, name=London, state=null, country=Country(id=9, name=United Kingdom)), City(id=2, name=Melbourne, state=Victoria, country=Country(id=1, name=Australia)), City(id=7, name=Miami, state=Florida, country=Country(id=3, name=USA)), City(id=4, name=Montreal, state=Quebec, country=Country(id=2, name=Canada)), City(id=8, name=New York, state=null, country=Country(id=3, name=USA)), City(id=12, name=Paris, state=null, country=Country(id=6, name=France)), City(id=11, name=Prague, state=null, country=Country(id=5, name=Czech Republic)), City(id=9, name=San Francisco, state=California, country=Country(id=3, name=USA)), City(id=3, name=Sydney, state=New South Wales, country=Country(id=1, name=Australia)), City(id=10, name=Tokyo, state=null, country=Country(id=4, name=Japan)) ].
Finally, it's time to move to our examples. Let's start with a simple isSorted method.
AssertJ framework provides the isSorted method in order to verify values that implement the Comparable interface, and these values are in a natural order. The simplest usage can be seen in the dummyAscendingSorting test as:
Assert the correct order with isSorted method (line 5).
Java @Test void dummyAscendingSorting() { var cities = [website]"Atlanta", "London", "Tokyo"); assertThat(cities).isSorted(); }.
Define a pagination request for loading data. Here, we request data sorted just by city name and the page with the size of 5 (line 5).
Load cities from cityRepository with the findAll method (line 5).
with the method (line 5). Assert the loaded data as: Check the number of cities returned by the search (line 10) -> to be equal to the requested page size. Extract a name attribute from the City entity (line 11). This is necessary as our City entity doesn't implement the Comparable interface. More details are covered at the end of this article. Assert the correct order with isSorted (line 12) -> this is our goal.
In some cases, we use descending order. The sorting assertion can be handled in a pretty similar way, but we need to use the isSortedAccordingTo method instead of the isSorted method. This method is used for the advanced sorting assertion.
The simplest assertion for values sorted in descending ways can be seen in the dummyDescendingSorting test. This is the same as the usage of the isSorted method, but this time, we need to use the already-mentioned isSortedAccordingTo method with the Collections.reverseOrder comparator.
Java import static [website]; @Test void dummyDescendingSorting() { assertThat([website]"Tokyo", "London", "Atlanta")).isSortedAccordingTo( reverseOrder() ); }.
Sometimes, we use sorting by multiple attributes. Therefore, we cannot use the simple approach shown in previous examples. For asserting sorting by multiple attributes, we need to have a comparator. This case is demonstrated in the sortingByCountryAndCityNames test as:
Define a pagination request with ascending sorting first by the country name and then by the city name (line 4). Now, we use a higher page size in order to load all available data.
Assert the loaded data as: Assert the correct order by the country name (line 4) with the custom comparator implemented in the getCountryNameComparator method (lines 13-15). Assert the correct order by the city name (line 10) simply by providing the desired function to the Comparator.thenComparing method.
Java @Test void sortingByCountryAndCityNames() { var countryNameSorting = City_.COUNTRY + "." + [website]; var pageable = [website], 15, ASC, countryNameSorting, [website]; Page page = cityRepository.findAll(pageable); assertThat(page.getContent()) .isSortedAccordingTo( getCountryNameComparator() .thenComparing( City::getName )); } private Comparator getCountryNameComparator() { return ( c1, c2 ) -> c1.getCountry().getName().compareTo(c2.getCountry().getName()); }.
In order to promote your understanding, the data by Spring Data JPA loaded in the sortingByCountryAndCityNames test is listed below as:
Plain Text [ City(id=1, name=Brisbane, state=Queensland, country=Country(id=1, name=Australia)), City(id=2, name=Melbourne, state=Victoria, country=Country(id=1, name=Australia)), City(id=3, name=Sydney, state=New South Wales, country=Country(id=1, name=Australia)), City(id=4, name=Montreal, state=Quebec, country=Country(id=2, name=Canada)), City(id=11, name=Prague, state=null, country=Country(id=5, name=Czech Republic)), City(id=12, name=Paris, state=null, country=Country(id=6, name=France)), City(id=10, name=Tokyo, state=null, country=Country(id=4, name=Japan)), City(id=13, name=Barcelona, state=Catalunya, country=Country(id=7, name=Spain)), City(id=14, name=Bern, state=null, country=Country(id=8, name=Switzerland)), City(id=5, name=Atlanta, state=Georgia, country=Country(id=3, name=USA)), City(id=6, name=Chicago, state=Illionis, country=Country(id=3, name=USA)), City(id=7, name=Miami, state=Florida, country=Country(id=3, name=USA)), City(id=8, name=New York, state=null, country=Country(id=3, name=USA)), City(id=9, name=San Francisco, state=California, country=Country(id=3, name=USA)), City(id=15, name=London, state=null, country=Country(id=9, name=United Kingdom)) ].
Some data might contain a null value, and we need to deal with it. This case is covered in the dummyAscendingSortingWithNull test as:
Define data with null value in the beginning (line 6).
value in the beginning (line 6). Assert null value in the beginning with Comparator.nullsFirst comparator and the ascending order by using Comparator.naturalOrder comparator.
Java import static [website]; import static [website]; @Test void dummyAscendingSortingWithNull() { assertThat([website] String[] { null, "London", "Tokyo" })) .isSortedAccordingTo(nullsFirst(naturalOrder())); }.
It is also possible to receive a null at the end instead of the beginning. Let's see this case in our last example.
Our last example demonstrates a more complex scenario. Our goal is to verify the order of our data sorted in descending and case-insensitive order. Additionally, this data contains null values. The simple usage is in the dummyDescendingSortingWithNull test as:
Assert the correct order with isSortedAccordingTo (line 8) and Comparator.nullsLast – to check that nulls are at the end -> as we have descending sorting, Collections.reverseOrder – to check the descending order and String.CASE_INSENSITIVE_ORDER – to compare values ignoring the case sensitivity.
Java import static [website]; import static [website]; import static String.CASE_INSENSITIVE_ORDER; @Test void dummyDescendingSortingWithNull() { assertThat([website] String[] { "London", "atlanta", "Alabama", null})) .isSortedAccordingTo(nullsLast(reverseOrder(CASE_INSENSITIVE_ORDER))); }.
When dealing with sorting, it's easy to forget we can apply sorting functions only to instances implementing the Comparable interface. In our case, the City entity doesn't implement this interface. The appropriate comparator depends on our sorting. Therefore, the comparator can be different for every sortable attribute or their combinations. Let's demonstrate this situation from our first example by the failByNotProvidingCorrectComparator test as:
Java import static [website]; @Test void failByNotProvidingCorrectComparator() { var pageable = [website], 5, ASC, [website]; Page page = cityRepository.findAll(pageable); assertThat(page.getContent()) .hasSize(5) // .map(City::getName) .isSorted(); }.
We get the some elements are not mutually comparable in group error when the map function is commented out (line 11).
Such simplification is wrong, but it can happen from time to time when we try to simplify our code.
First, the article explained the basics of sorting with the isSorted method. Next, sorting assertions for data in reverse order and sorting by two criteria using the custom comparator were demonstrated.
After that, the sorting for data with null values was covered. Finally, the pitfall related to the misuse of sorting assertions provided by the AssertJ framework was explained.
The complete source code presented above is available in my GitHub repository.
In the world of programming, errors are inevitable. Whether caused by unexpected inputs, system issues, or edge cases, they have the......
One of the key principles of writing a good data pipeline is ensuring accurate data is loaded into the target table. We have no control over......
The Azure AI Agent Service is Microsoft’s enterprise-grade implementation of AI agents. It empowers developers to build, deploy, and scale sophisticat......
Mastering JavaScript Proxies and Reflect for Real-World Use
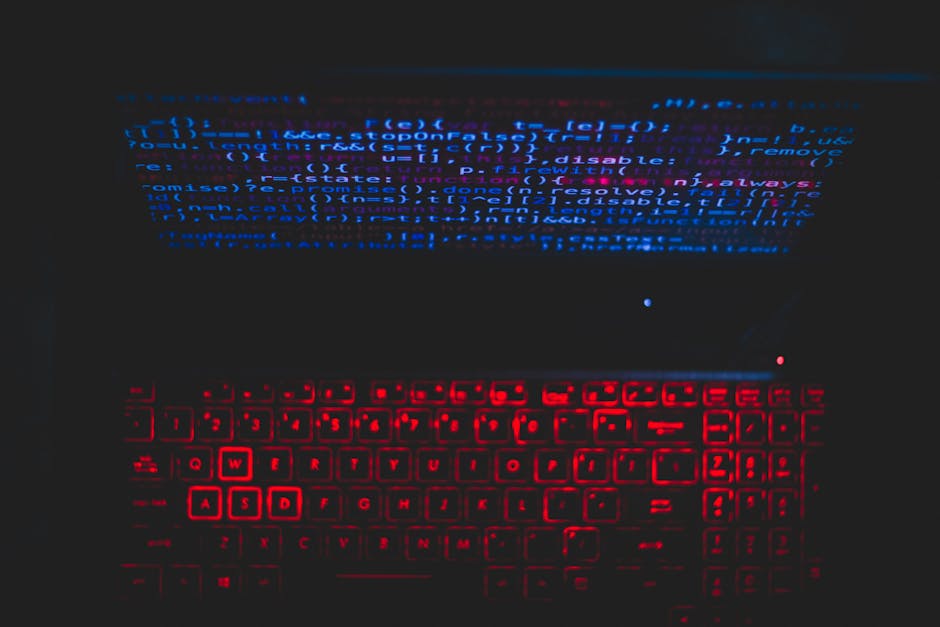
JavaScript is always evolving, with new tools and patterns continually emerging to help developers write more effective, more powerful code. Two game-changing yet often underused aspects are Proxy and the Reflect API. These tools allow you to intercept and manipulate the way objects behave, allowing for advanced functionality like custom property access, validation and more.
Proxies and Reflect aren’t just academic programming concepts; they solve real problems that developers face every day. Whether it’s logging interactions with objects, enforcing data validation rules or creating reactive systems like those used in modern frameworks, these tools offer practical solutions to common challenges in software development.
By following this guide, you’ll gain a deeper understanding of how Proxies and Reflect work, see them in action with clear examples and discover how to use them to create cleaner, more dynamic and more efficient applications.
A JavaScript Proxy acts as a wrapper around an object, intercepting operations like property access, assignment and function invocation. It allows developers to define custom behavior for these operations using “traps” — handler functions that override default object behavior.
const target = { message: "Hello, Proxy!" }; const handler = { get: (obj, prop) => { [website]`Accessed property: ${prop}`); return obj[prop]; } }; const proxy = new Proxy(target, handler); [website]; // Logs: Accessed property: message 1 2 3 4 5 6 7 8 9 10 11 12 const target = { message: "Hello, Proxy!" }; const handler = { get: ( obj , prop ) = > { console . log (` Accessed property: ${ prop }`); return obj [ prop ]; } }; const proxy = new Proxy ( target , handler ); console . log ( proxy . message ); // Logs: Accessed property: message.
For instance, look at the above code. The get trap intercepts property access, logging the accessed property name before returning its value.
The Reflect API complements Proxies by providing a set of static methods to perform common object operations, such as [website] , [website] and [website] . It ensures consistent behavior when traps override default operations. Using Reflect methods within traps can help maintain standard object behavior while adding custom logic.
const handler = { get: (obj, prop) => { [website]`Property accessed: ${prop}`); return [website], prop); // Maintains default behavior } }; 1 2 3 4 5 6 const handler = { get: ( obj , prop ) = > { console . log (` Property accessed: ${ prop }`); return Reflect . get ( obj , prop ); // Maintains default behavior } };
Reflect allows you to seamlessly integrate custom and default behavior within your Proxy traps.
Here are some real-world use cases where they could come in handy:
1. Logging property access and updates: Proxies can provide insightful logging for debugging or auditing application state.
const logger = new Proxy({}, { set: (obj, prop, value) => { [website]`Property ${prop} set to ${value}`); return [website], prop, value); } }); [website] = "JavaScript"; logger.version = "ES6"; // Logs: // Property name set to JavaScript // Property version set to ES6 1 2 3 4 5 6 7 8 9 10 11 12 const logger = new Proxy ({}, { set: ( obj , prop , value ) = > { console . log (` Property ${ prop } set to ${ value }`); return Reflect . set ( obj , prop , value ); } }); logger . name = "JavaScript" ; logger . version = "ES6" ; // Logs: // Property name set to JavaScript // Property version set to ES6.
In the above code, the first argument to the Proxy constructor is the target object, which in this case is an empty object ({}) . The Proxy wraps this target, intercepting operations performed on it. The second argument is the handler object, which defines traps or hooks to customize behavior for specific operations. In this example, the set trap is used to intercept property assignments. The set trap is a function that takes three arguments: the original target object ( obj ), the property being set ( prop ), and the new value assigned to that property ( value ). This allows developers to define custom behavior for property assignments while still preserving the default functionality if needed.
The trap then performs two tasks: first, it logs the property name and value being set.
[website] Property ${prop} set to ${value} );
Then it ensures the property is set on the target object using [website] . Without [website] , the property would not be stored in the object.
return [website], prop, value); [website] = "JavaScript"; logger.version = "ES6"; 1 2 3 4 return Reflect . set ( obj , prop , value ); logger . name = "JavaScript" ; logger . version = "ES6" ;
[website] = "JavaScript" then triggers the set trap, which logs the property name set to JavaScript. Then, [website] ensures the name property is added to the logger object.
logger.version = "ES6" does the same, logging the property version set to ES6.
The key takeaways in this scenario include dynamic interception where proxies allow you to dynamically intercept and customize object behavior. In this case, all property changes are logged; Reflect API provides a way to perform default behavior (such as setting a property) without directly manipulating the object, ensuring cleaner and safer code. This pattern is useful for debugging, logging or adding constraints, such as validation before setting a value.
2. Input validation: Proxies can enforce constraints on objects, ensuring data integrity.
const validator = new Proxy({}, { set: (obj, prop, value) => { if (prop === "age" && (value < 0 || value > 120)) { throw new Error("Invalid age"); } return [website], prop, value); } }); [website] = 25; // Works [website] = -5; // Throws: Invalid age 1 2 3 4 5 6 7 8 9 10 11 const validator = new Proxy ({}, { set: ( obj , prop , value ) = > { if ( prop === "age" && (value < 0 || value > 120)) { throw new Error("Invalid age"); } return Reflect . set ( obj , prop , value ); } }); validator . age = 25 ; // Works validator . age = - 5 ; // Throws: Invalid age.
In the above code, validation logic is first executed before the property is set to the new value to ensure that only correct data is passed. In this particular case, the validator proxy is designed to enforce validation rules on a target object (an empty object {} in this case). It uses the set trap to intercept property assignments. Whenever a property is set, the trap checks if the property being modified is age . If it is, it ensures the value is within the valid range (0 to 120). If the value is outside this range, an error is thrown with the message “Invalid age.” If the validation passes, the [website] set method is called to complete the property assignment and preserve the default behavior. This approach adds a layer of logic to ensure data integrity for the age property.
3. Data binding for reactive UIs: Proxies simplify building reactive systems by detecting changes to data and triggering updates.
const state = new Proxy({}, { set: (obj, prop, value) => { [website]`State changed: ${prop} = ${value}`); document.getElementById(prop).innerText = value; return [website], prop, value); } }); state.username = "John"; // Updates a DOM element with id="username" 1 2 3 4 5 6 7 8 9 const state = new Proxy ({}, { set: ( obj , prop , value ) = > { console . log (` State changed: ${ prop } = ${ value }`); document . getElementById ( prop ). innerText = value ; return Reflect . set ( obj , prop , value ); } }); state . username = "John" ; // Updates a DOM element with id="username"
The above code creates a state object using a Proxy to track and dynamically modification UI changes when properties are modified. The set trap intercepts property assignments, logging the property name and its new value to the console. Additionally, it updates the text content of a Document Object Model (DOM) element whose id matches the property name being modified, reflecting the new value in the UI. Finally, it calls [website] to perform the actual property assignment, maintaining default object behavior. For example, when state.username is set to John , it logs the change and updates the content of the DOM element with id="username" to display John .
The use of proxies with the Reflect API offers several key advantages that improve the overall design and maintainability of code. One of the primary benefits is cleaner logic, as the Reflect API streamlines the implementation of traps, such as get , set and deleteProperty , by providing a standardized and predictable interface. This reduces the need for repetitive boilerplate code, making the logic behind proxy behavior more concise and easier to follow.
Moreover, proxies in conjunction with Reflect support dynamic behavior, meaning that the proxy can adapt to changing requirements or states without altering the underlying object. This dynamic adaptability allows you to introduce additional behavior or validation logic at runtime, such as logging access to properties or modifying data before it’s written, without directly modifying the original object or class.
Finally, proxies with Reflect enable centralized control of certain aspects of your application. For instance, rather than scattering validation or logging logic throughout the codebase, you can centralize it in a single handler, which simplifies debugging and ongoing maintenance. This centralization makes it easier to monitor and control interactions with objects, ensuring that behaviors are consistent and easy to modify, reducing complexity and improving the overall robustness of your application.
When working with JavaScript Proxies and the Reflect API, following best practices is key to writing efficient, secure and maintainable code. Reflect is especially useful for keeping things consistent. By using it to invoke default behaviors alongside your custom logic, you ensure your proxy behaves predictably, reducing the risk of unexpected side effects.
Performance is another critical factor. Overusing traps, particularly in frequently accessed properties or methods, can slow down your application. To avoid this, keep trap usage minimal in performance-critical areas and focus on optimizing their implementation when necessary.
Security is just as essential. Always validate inputs and outputs within your traps, and avoid exposing sensitive information through your handlers. Careful validation and controlling data access help prevent your proxies from introducing security vulnerabilities.
By sticking to these best practices, you can create solutions that are efficient, reliable and secure.
JavaScript Proxies and the Reflect API offer incredible control over object behavior, unlocking new ways to solve common development challenges. Whether you’re building debugging tools, enforcing validation or creating reactive UIs, these attributes can streamline your code while adding powerful functionality. With real-world use cases like logging, validation and data binding, learning to master Proxies and Reflect can take your JavaScript skills to the next level and make your applications more dynamic and resilient.
If you’re eager to expand your knowledge about APIs and take your expertise to the next level, read Andela’s article “Overcoming the Challenges of Working With a Mobile FinTech API,” featuring additional insights into dynamic JavaScript elements.
Amazon Elastic MapReduce (EMR) is a platform to process and analyze big data. Traditional EMR runs on a cluster of Amazon EC2 instances managed by AWS......
AI-driven data trends in Indian governance in 2025 are revolutionizing decision-making, enhancing efficiency, and improving public servi......
I’ve been a software engineer for a little over a decade now, and I like to think I’m a pretty organized person. I have a system for everything, and t......
Rust Code Generation: A Complete Guide to Automated Development Tools and Macros
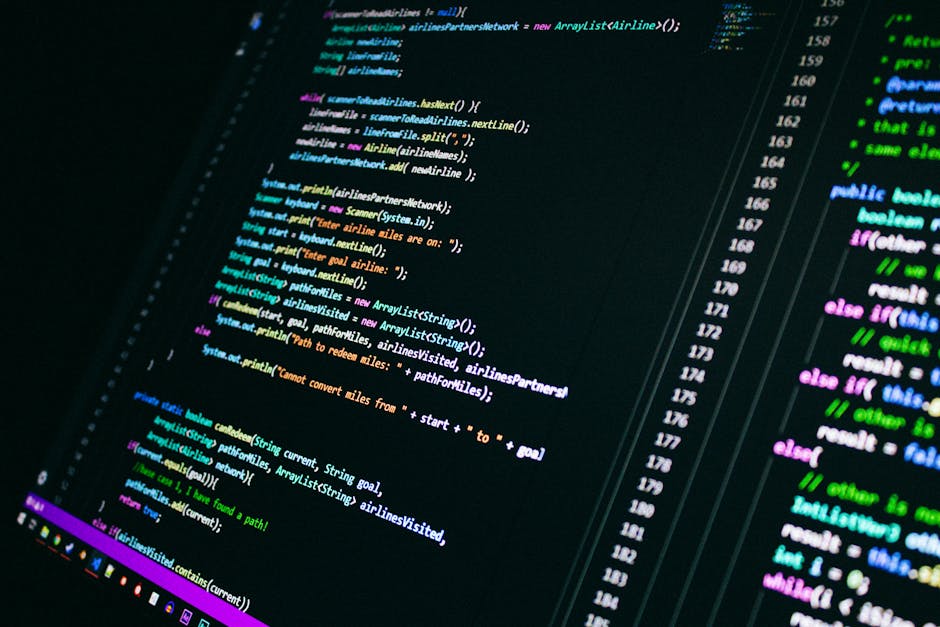
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Rust's code generation ecosystem represents a powerful feature set that transforms how developers write and maintain code. The language provides sophisticated tools that automate repetitive tasks while ensuring type safety and performance optimization.
At the core of Rust's code generation lies the derive macro system, a feature that streamlines the implementation of common traits. Rather than writing boilerplate code manually, developers can annotate their types with derive attributes to generate standardized implementations automatically.
#[derive(Debug, Clone, PartialEq)] struct Product { id : u32 , name : String , price : f64 } Enter fullscreen mode Exit fullscreen mode.
The [website] script serves as a pre-compilation code generator. This system runs before the main compilation process, enabling dynamic code generation based on external factors. It's particularly useful for generating code from protocol definitions, database schemas, or system-specific configurations.
// [website] fn main () { println! ( "cargo:rerun-if-changed=src/[website]" ); let schema = std :: fs :: read_to_string ( "src/[website]" ) .unwrap (); generate_code_from_schema ( & schema ); } Enter fullscreen mode Exit fullscreen mode.
Custom derive macros extend the code generation capabilities further. These macros analyze type definitions and generate specialized implementations based on the type's structure and attributes. They're instrumental in implementing complex patterns like serialization, builder patterns, and data validation.
use proc_macro :: TokenStream ; #[proc_macro_derive(Builder)] pub fn derive_builder ( input : TokenStream ) -> TokenStream { let ast = syn :: parse ( input ) .unwrap (); implement_builder ( & ast ) } Enter fullscreen mode Exit fullscreen mode.
The serde framework demonstrates the power of Rust's code generation. It automatically implements serialization and deserialization for custom types, handling complex data structures with minimal manual intervention.
#[derive(Serialize, Deserialize)] struct Configuration { database_url : String , max_connections : u32 , timeout_seconds : u64 , features : Vec < String > } Enter fullscreen mode Exit fullscreen mode.
Procedural macros offer the most flexible code generation capabilities. They can create entirely new code structures, modify existing code, and implement complex patterns. This makes them valuable for creating domain-specific languages and reducing repetitive code patterns.
#[proc_macro] pub fn create_api_endpoints ( input : TokenStream ) -> TokenStream { let routes = parse_route_definitions ( input ); generate_endpoint_implementations ( & routes ) } Enter fullscreen mode Exit fullscreen mode.
The Builder pattern, commonly implemented through code generation, creates fluent interfaces for complex object construction. This pattern ensures type safety while providing a readable and maintainable API.
#[derive(Builder)] struct HttpClient { base_url : String , timeout : Duration , retry_count : u32 , headers : HashMap < String , String > } let client = HttpClientBuilder :: default () .base_url ( "[website]" ) .timeout ( Duration :: from_secs ( 30 )) .retry_count ( 3 ) .headers ( default_headers ()) .build () .unwrap (); Enter fullscreen mode Exit fullscreen mode.
Error handling patterns benefit from code generation through custom error types. These generated implementations reduce boilerplate while maintaining type safety and proper error propagation.
#[derive(Error, Debug)] enum ServiceError { #[error( "Database error: {0}" )] Database ( #[from] sqlx :: Error ), #[error( "Invalid input: {0}" )] ValidationError ( String ), #[error( "Network error: {0}" )] NetworkError ( #[from] reqwest :: Error ) } Enter fullscreen mode Exit fullscreen mode.
Testing frameworks utilize code generation to create comprehensive test suites. This includes generating test cases from data files and implementing common testing patterns automatically.
#[derive(TestCases)] #[test_resource( "[website]" )] struct ValidationTests { input : String , expected_output : Result < User , ValidationError > } Enter fullscreen mode Exit fullscreen mode.
The type system interfaces with code generation to implement trait bounds and generic constraints. This ensures type safety while reducing the amount of manual implementation required.
#[derive(AsRef, DerefMut)] struct Wrapper < T > ( Vec < T > ); Enter fullscreen mode Exit fullscreen mode.
Configuration management benefits from code generation through automated parsing and validation of configuration files. This ensures type safety and proper error handling for configuration values.
#[derive(Deserialize, Validate)] struct AppConfig { #[validate(range(min = 1024 , max = 65535 ))] port : u16 , #[validate(url)] api_endpoint : String , #[validate(email)] admin_email : String } Enter fullscreen mode Exit fullscreen mode.
Database interactions often utilize code generation to create type-safe queries and model definitions. This ensures consistency between the database schema and application code.
#[derive(Queryable, Insertable)] #[table_name = "users" ] struct User { id : i32 , username : String , email : String , created_at : DateTime < Utc > } Enter fullscreen mode Exit fullscreen mode.
API client generation automates the creation of type-safe client libraries from API specifications. This ensures consistency between the API definition and client code.
#[derive(OpenApi)] #[openapi(spec = "[website]" )] struct ApiClient ; Enter fullscreen mode Exit fullscreen mode.
Code generation in Rust extends to async code as well. The async-trait macro generates appropriate implementations for async traits, handling the complexity of async fn in traits.
#[async_trait] trait DataStore { async fn get_user ( & self , id : UserId ) -> Result < User , Error > ; async fn save_user ( & self , user : & User ) -> Result < (), Error > ; } Enter fullscreen mode Exit fullscreen mode.
Command-line interface generation simplifies the creation of CLI applications. The clap derive macro generates argument parsing code from struct definitions.
#[derive(Parser)] struct Cli { #[clap(short, long)] config : PathBuf , #[clap(short, long, default_value = "info" )] log_level : String , #[clap(subcommand)] command : Commands } Enter fullscreen mode Exit fullscreen mode.
These code generation capabilities significantly enhance developer productivity while maintaining Rust's strong safety guarantees. They reduce the likelihood of errors in repetitive code patterns and enable the creation of sophisticated abstractions with minimal boilerplate.
The integration of these tools into the Rust ecosystem creates a powerful development environment where common patterns are implemented consistently and correctly. This allows developers to focus on business logic while relying on generated code for standard functionality.
101 Books is an AI-driven publishing organization co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools.
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva.
I'd like to build a GitHub repository to begin a new project for pulling data from the website [website] Who wants to join?
When working with MySQL in your Java applications, there are several layers at which you can optimize performance. In this post, I’ll cover key areas—......
AI-Driven Automation: AI will automate API lifecycle management, enhancing performance and security. API-First Development: Designing A......
Market Impact Analysis
Market Growth Trend
2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|
7.5% | 9.0% | 9.4% | 10.5% | 11.0% | 11.4% | 11.5% |
Quarterly Growth Rate
Q1 2024 | Q2 2024 | Q3 2024 | Q4 2024 |
---|---|---|---|
10.8% | 11.1% | 11.3% | 11.5% |
Market Segments and Growth Drivers
Segment | Market Share | Growth Rate |
---|---|---|
Enterprise Software | 38% | 10.8% |
Cloud Services | 31% | 17.5% |
Developer Tools | 14% | 9.3% |
Security Software | 12% | 13.2% |
Other Software | 5% | 7.5% |
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity:
Competitive Landscape Analysis
Company | Market Share |
---|---|
Microsoft | 22.6% |
Oracle | 14.8% |
SAP | 12.5% |
Salesforce | 9.7% |
Adobe | 8.3% |
Future Outlook and Predictions
The Demystifying Sorting Assertions landscape is evolving rapidly, driven by technological advancements, changing threat vectors, and shifting business requirements. Based on current trends and expert analyses, we can anticipate several significant developments across different time horizons:
Year-by-Year Technology Evolution
Based on current trajectory and expert analyses, we can project the following development timeline:
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity, influencing adoption timelines and investment priorities:
Innovation Trigger
- Generative AI for specialized domains
- Blockchain for supply chain verification
Peak of Inflated Expectations
- Digital twins for business processes
- Quantum-resistant cryptography
Trough of Disillusionment
- Consumer AR/VR applications
- General-purpose blockchain
Slope of Enlightenment
- AI-driven analytics
- Edge computing
Plateau of Productivity
- Cloud infrastructure
- Mobile applications
Technology Evolution Timeline
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
Expert Perspectives
Leading experts in the software dev sector provide diverse perspectives on how the landscape will evolve over the coming years:
"Technology transformation will continue to accelerate, creating both challenges and opportunities."
— Industry Expert
"Organizations must balance innovation with practical implementation to achieve meaningful results."
— Technology Analyst
"The most successful adopters will focus on business outcomes rather than technology for its own sake."
— Research Director
Areas of Expert Consensus
- Acceleration of Innovation: The pace of technological evolution will continue to increase
- Practical Integration: Focus will shift from proof-of-concept to operational deployment
- Human-Technology Partnership: Most effective implementations will optimize human-machine collaboration
- Regulatory Influence: Regulatory frameworks will increasingly shape technology development
Short-Term Outlook (1-2 Years)
In the immediate future, organizations will focus on implementing and optimizing currently available technologies to address pressing software dev challenges:
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
These developments will be characterized by incremental improvements to existing frameworks rather than revolutionary changes, with emphasis on practical deployment and measurable outcomes.
Mid-Term Outlook (3-5 Years)
As technologies mature and organizations adapt, more substantial transformations will emerge in how security is approached and implemented:
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
This period will see significant changes in security architecture and operational models, with increasing automation and integration between previously siloed security functions. Organizations will shift from reactive to proactive security postures.
Long-Term Outlook (5+ Years)
Looking further ahead, more fundamental shifts will reshape how cybersecurity is conceptualized and implemented across digital ecosystems:
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
These long-term developments will likely require significant technical breakthroughs, new regulatory frameworks, and evolution in how organizations approach security as a fundamental business function rather than a technical discipline.
Key Risk Factors and Uncertainties
Several critical factors could significantly impact the trajectory of software dev evolution:
Organizations should monitor these factors closely and develop contingency strategies to mitigate potential negative impacts on technology implementation timelines.
Alternative Future Scenarios
The evolution of technology can follow different paths depending on various factors including regulatory developments, investment trends, technological breakthroughs, and market adoption. We analyze three potential scenarios:
Optimistic Scenario
Rapid adoption of advanced technologies with significant business impact
Key Drivers: Supportive regulatory environment, significant research breakthroughs, strong market incentives, and rapid user adoption.
Probability: 25-30%
Base Case Scenario
Measured implementation with incremental improvements
Key Drivers: Balanced regulatory approach, steady technological progress, and selective implementation based on clear ROI.
Probability: 50-60%
Conservative Scenario
Technical and organizational barriers limiting effective adoption
Key Drivers: Restrictive regulations, technical limitations, implementation challenges, and risk-averse organizational cultures.
Probability: 15-20%
Scenario Comparison Matrix
Factor | Optimistic | Base Case | Conservative |
---|---|---|---|
Implementation Timeline | Accelerated | Steady | Delayed |
Market Adoption | Widespread | Selective | Limited |
Technology Evolution | Rapid | Progressive | Incremental |
Regulatory Environment | Supportive | Balanced | Restrictive |
Business Impact | Transformative | Significant | Modest |
Transformational Impact
Technology becoming increasingly embedded in all aspects of business operations. This evolution will necessitate significant changes in organizational structures, talent development, and strategic planning processes.
The convergence of multiple technological trends—including artificial intelligence, quantum computing, and ubiquitous connectivity—will create both unprecedented security challenges and innovative defensive capabilities.
Implementation Challenges
Technical complexity and organizational readiness remain key challenges. Organizations will need to develop comprehensive change management strategies to successfully navigate these transitions.
Regulatory uncertainty, particularly around emerging technologies like AI in security applications, will require flexible security architectures that can adapt to evolving compliance requirements.
Key Innovations to Watch
Artificial intelligence, distributed systems, and automation technologies leading innovation. Organizations should monitor these developments closely to maintain competitive advantages and effective security postures.
Strategic investments in research partnerships, technology pilots, and talent development will position forward-thinking organizations to leverage these innovations early in their development cycle.
Technical Glossary
Key technical terms and definitions to help understand the technologies discussed in this article.
Understanding the following technical concepts is essential for grasping the full implications of the security threats and defensive measures discussed in this article. These definitions provide context for both technical and non-technical readers.