Resolving Duplicate Key Issues in React Infinite Scroll with MongoDB Sort Behavior - Related to duplicate, performance, scroll, arch, optimization:
Install Arch with swapfile baby.
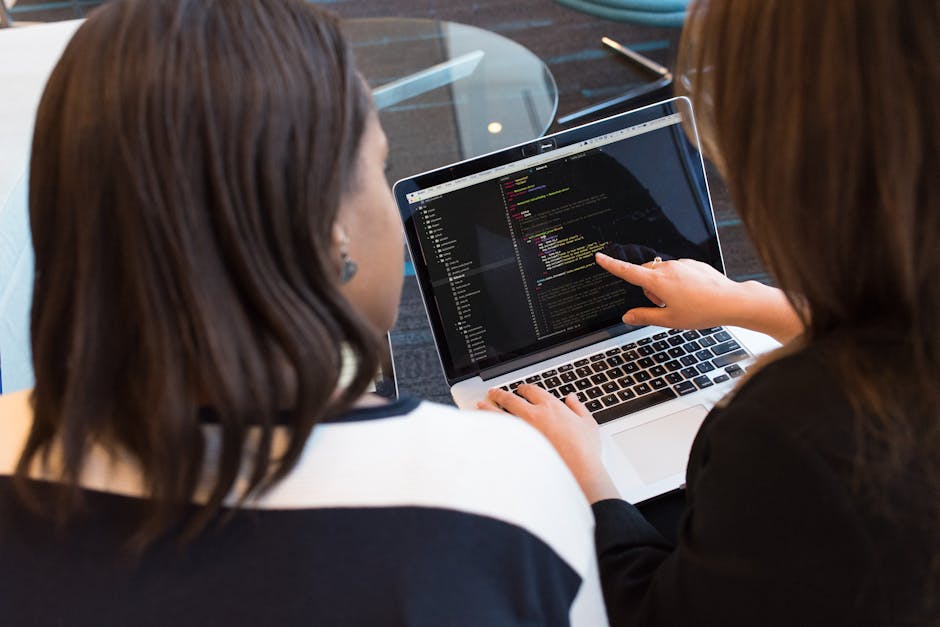
Hi, let's get started with our install. Download the ISO and boot it however you want.
# Connect to your WiFi (replace with your network) iwctl station wlan0 connect Enter fullscreen mode Exit fullscreen mode.
# Ping a website to check connectivity ping [website] Enter fullscreen mode Exit fullscreen mode.
# Set network time protocol timedatectl set-ntp true Enter fullscreen mode Exit fullscreen mode.
# List disks to identify your target disk (mine is /dev/sda) lsblk Enter fullscreen mode Exit fullscreen mode.
# Partition disk /dev/sda: fdisk /dev/sda # Inside fdisk, input the following: # g -> Create a new GPT partition table # n -> Create new partition (EFI) # -> Accept default partition number # -> Accept default first sector # -> Enter "+512M" for size # n -> Create new partition (Linux filesystem) # -> Accept defaults to use the remaining space # w -> Write changes and exit Enter fullscreen mode Exit fullscreen mode.
# Format EFI partition as FAT32 and Linux partition as ext4 [website] -F32 /dev/sda1 [website] /dev/sda2 Enter fullscreen mode Exit fullscreen mode.
# Mount the Linux partition and create/mount the boot directory for EFI mount /dev/sda2 /mnt mkdir -p /mnt/boot mount /dev/sda1 /mnt/boot Enter fullscreen mode Exit fullscreen mode.
# Install base system, Linux kernel, firmware, and vim pacstrap /mnt base linux linux-firmware vim Enter fullscreen mode Exit fullscreen mode.
# Generate filesystem table genfstab -U /mnt >> /mnt/etc/fstab cat /mnt/etc/fstab Enter fullscreen mode Exit fullscreen mode.
# Enter the new system environment arch-chroot /mnt Enter fullscreen mode Exit fullscreen mode.
# Create and activate a 16GB swapfile fallocate -l 16GB /swapfile chmod 600 /swapfile mkswap /swapfile swapon /swapfile Enter fullscreen mode Exit fullscreen mode.
# Open fstab and add the swapfile entry at the end vim /etc/fstab # Add: /swapfile none swap defaults 0 0 Enter fullscreen mode Exit fullscreen mode.
# Link timezone and sync hardware clock ln -sf /usr/share/zoneinfo/Asia/Kathmandu /etc/localtime hwclock --systohc Enter fullscreen mode Exit fullscreen mode.
# Edit [website] to uncomment [website] and generate locale vim /etc/[website] locale-gen echo "[website]" > /etc/[website] Enter fullscreen mode Exit fullscreen mode.
# Set hostname to "arch" echo "arch" > /etc/hostname Enter fullscreen mode Exit fullscreen mode.
# Edit /etc/hosts for proper hostname resolution vim /etc/hosts # Add the following: # [website] localhost # ::1 localhost # [website] arch.localdomain arch Enter fullscreen mode Exit fullscreen mode.
# Set the root password passwd Enter fullscreen mode Exit fullscreen mode.
# Install systemd-boot on the EFI partition bootctl install # Create loader configuration vim /boot/loader/[website] # Add: # default arch # timeout 3 # editor no # Create boot entry for Arch Linux vim /boot/loader/entries/[website] # Add: # title Arch Linux # linux /vmlinuz-linux # initrd /[website] # options root=UUID= rw # Get UUID: blkid /dev/sda2 Enter fullscreen mode Exit fullscreen mode.
19. Install Additional Packages & Finalize.
# Install necessary packages pacman -S networkmanager network-manager-applet wpa_supplicant dialog os-prober mtools dosfstools base-devel linux-headers # Exit chroot, unmount all partitions, and reboot exit umount -a reboot Enter fullscreen mode Exit fullscreen mode.
# Use nmcli or your preferred network manager after reboot nmcli device wifi list nmcli device wifi connect password Enter fullscreen mode Exit fullscreen mode.
# Create a new user and add to the wheel group useradd -m -G wheel baby passwd baby # Allow wheel group sudo privileges EDITOR = vim visudo # Uncomment: %wheel ALL=(ALL) ALL Enter fullscreen mode Exit fullscreen mode.
# For Intel: sudo pacman -S xf86-video-intel # For AMD: sudo pacman -S xf86-video-amdgpu # For NVIDIA: sudo pacman -S nvidia nvidia-utils Enter fullscreen mode Exit fullscreen mode.
// By default, ALSA provides basic audio support. // For a modern audio stack, install PipeWire. # Install PipeWire and its PulseAudio replacement sudo pacman -S pipewire pipewire-pulse pipewire-alsa // Most apps will use PipeWire automatically. // Optionally, install a volume control ([website], pavucontrol) if needed. sudo pacman -S pavucontrol // That's it for audio! Enter fullscreen mode Exit fullscreen mode.
# Install Hyprland (Wayland compositor) sudo pacman -S hyprland # For a login greeter, consider installing ly sudo pacman -S ly Enter fullscreen mode Exit fullscreen mode.
We're a place where coders share, stay up-to-date and grow their careers....
A little gem from Kevin Powell’s “HTML & of the Week” website, reminding us that using container queries opens up container query units for si......
We're a place where coders share, stay up-to-date and grow their careers....
Docker Performance Optimization: Real-World Strategies
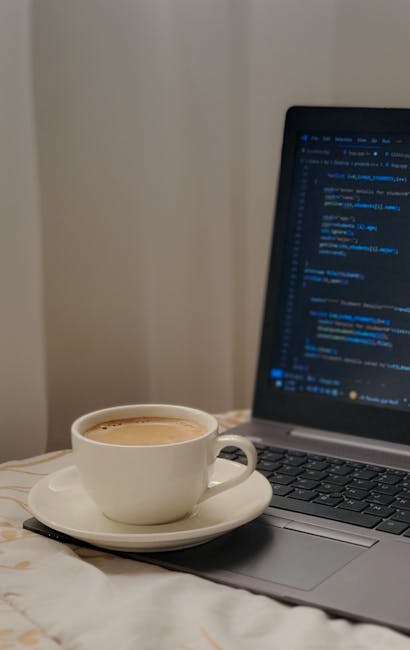
After optimizing containerized applications processing petabytes of data in fintech environments, I've learned that Docker performance isn't just about speed — it's about reliability, resource efficiency, and cost optimization. Let's dive into strategies that actually work in production.
The Performance Journey: Common Scenarios and Solutions.
Have you ever seen your container CPU usage spike to 100% for no apparent reason? We can fix that with this code below:
Shell # Quick diagnosis script #!/bin/bash container_id=$1 echo "CPU Usage Analysis" docker stats --no-stream $container_id echo "Top Processes Inside Container" docker exec $container_id top -bn1 echo "Hot CPU Functions" docker exec $container_id perf top -a.
This script provides three levels of CPU analysis:
docker stats – exhibits real-time CPU usage percentage and other resource metrics.
– presents real-time CPU usage percentage and other resource metrics top -bn1 – lists all processes running inside the container, sorted by CPU usage.
– lists all processes running inside the container, sorted by CPU usage perf top -a – identifies specific functions consuming CPU cycles.
After identifying CPU bottlenecks, here's how to implement resource constraints and optimizations:
YAML services: cpu-optimized: deploy: resources: limits: cpus: '2' reservations: cpus: '1' environment: # JVM optimization (if using Java) JAVA_OPTS: > -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -XX:ParallelGCThreads=4 -XX:ConcGCThreads=2.
Limits the container to use maximum 2 CPU cores.
Optimizes Java applications by: Using the G1 garbage collector for advanced throughput Setting a maximum pause time of 200ms for garbage collection Configuring parallel and concurrent GC threads for optimal performance.
If you have a container with growing memory usage, here is your debugging toolkit:
Shell #!/bin/bash # [website] container_name=$1 echo "Memory Trend Analysis" while true; do docker stats --no-stream $container_name | \ awk '{print strftime("%H:%M:%S"), $4}' >> [website] sleep 10 done.
Logs timestamp and memory usage to [website].
Uses awk to format the output with timestamps.
Plain Text Before Optimization: - Base Memory: 750MB - Peak Memory: [website] - Memory Growth Rate: +100MB/hour After Optimization: - Base Memory: 256MB - Peak Memory: 512MB - Memory Growth Rate: +5MB/hour - Memory Use Pattern: Stable with regular GC.
If your container is taking ages to start, we can fix it with the code below:
Dockerfile # Before: 45s startup time FROM openjdk:11 COPY . . RUN ./gradlew build # After: 12s startup time FROM openjdk:11-jre-slim as builder WORKDIR /app COPY [website] [website] ./ COPY src ./src RUN ./gradlew build --parallel --daemon FROM openjdk:11-jre-slim COPY --from=builder /app/build/libs/*.jar [website] # Enable JVM tiered compilation for faster startup ENTRYPOINT ["java", "-XX:+TieredCompilation", "-XX:TieredStopAtLevel=1", "-jar", "[website]"].
Multi-stage build reduces final image size.
Copying only necessary files for building.
Enabling parallel builds with Gradle daemon.
JVM tiered compilation optimizations: -XX:+TieredCompilation – enables tiered compilation -XX:TieredStopAtLevel=1 – stops at first tier for faster startup.
Real-World Performance Metrics Dashboard.
Here's a Grafana dashboard query that will give you the full picture:
YAML # [website] scrape_configs: - job_name: 'docker-metrics' static_configs: - targets: ['localhost:9323'] metrics_path: /metrics metric_relabel_configs: - source_labels: [container_name] regex: '^/.+' target_label: container_name replacement: '$1'
Sets up a scrape job named 'docker-metrics'
Targets the Docker metrics endpoint on localhost:9323.
Configures metric relabeling to clean up container names.
Collects all Docker engine and container metrics.
Plain Text Container Health Metrics: Response Time (p95): < 200ms CPU Usage: < 80% Memory Usage: < 70% Container Restarts: 0 in 24h Network Latency: < 50ms Warning Signals: Response Time > 500ms CPU Usage > 85% Memory Usage > 80% Container Restarts > 2 in 24h Network Latency > 100ms.
Here's my go-to performance investigation toolkit:
Shell #!/bin/bash # [website] container_name=$1 echo "Container Performance Analysis" # Check base stats docker stats --no-stream $container_name # Network connections echo "Network Connections" docker exec $container_name netstat -tan # File system usage echo "File System Usage" docker exec $container_name df -h # Process tree echo "Process Tree" docker exec $container_name pstree -p # I/O stats echo "I/O Statistics" docker exec $container_name iostat.
Network connection status and statistics.
Here are some real numbers from a recent optimization project:
Plain Text API Service Performance: Before → After - Requests/sec: 1,200 → 3,500 - Latency (p95): 250ms → 85ms - CPU Usage: 85% → 45% - Memory: [website] → 512MB Database Container: Before → After - Query Response: 180ms → 45ms - Connection Pool Usage: 95% → 60% - I/O Wait: 15% → 3% - Cache Hit Ratio: 75% → 95%.
The Performance Troubleshooting Playbook.
Shell # Quick startup analysis docker events --filter 'type=container' --filter 'event=start' docker logs --since 5m container_name.
The first command ( docker events ) monitors real-time container events, specifically filtered for: type=container – only show container-related events event=start – focus on container startup events.
) monitors real-time container events, specifically filtered for: The second command ( docker logs ) retrieves logs from the last 5 minutes for the specified container.
Container fails to start or starts slowly.
Investigating container startup dependencies.
Identifying startup-time configuration issues.
Shell # Network debugging toolkit docker run --rm \ --net container:target_container \ nicolaka/netshoot \ iperf -c iperf-server.
--rm – automatically remove the container when it exits.
– automatically remove the container when it exits --net container:target_container – share the network namespace with the target container.
– share the network namespace with the target container nicolaka/netshoot – a specialized networking troubleshooting container image.
– a specialized networking troubleshooting container image iperf -c iperf-server – network performance testing tool -c – run in client mode iperf-server – target server to test against.
Shell # Resource monitoring docker run --rm \ --pid container:target_container \ --net container:target_container \ nicolaka/netshoot \ htop.
--pid container:target_container – share the process namespace with target container.
– share the process namespace with target container --net container:target_container – share the network namespace.
– share the network namespace htop – interactive process viewer and system monitor.
YAML services: app: tmpfs: - /tmp:rw,noexec,nosuid,size=1g.
Mounts a tmpfs (in-memory filesystem) at /tmp.
Allocates 1GB of RAM for temporary storage.
Improves I/O performance for temporary files.
Options explained: rw – read-write access noexec – prevents execution of binaries nosuid – disables SUID/SGID bits.
Shell echo "[website]" >> /etc/[website] echo "[website]" >> /etc/[website].
Enable Fair Queuing scheduler for advanced latency.
Activate BBR congestion control algorithm.
Dockerfile FROM [website] AS builder WORKDIR /app COPY . . RUN CGO_ENABLED=0 go build -o server FROM [website] COPY --from=builder /app/server / CMD ["/server"].
Distroless base image for minimal attack surface.
Significant reduction in final image size.
Remember, Docker performance optimization is a more gradual process. Start with these metrics and tools, but always measure and adapt based on your specific needs. These strategies have helped me handle millions of transactions in production environments, and I'm confident they'll help you, too!
What Is Data Governance, and How Do Data Quality, Policies, and Procedures Strengthen It?
Data governance refers to the overall management of data av......
I'd like to build a GitHub repository to begin a new project for pulling data from the website [website] Who wants to join?
Coding to Figma UI design specifications can be time-consuming for frontend developers. A new artificial intelligence tool called AutoCode from WaveMa......
Resolving Duplicate Key Issues in React Infinite Scroll with MongoDB Sort Behavior
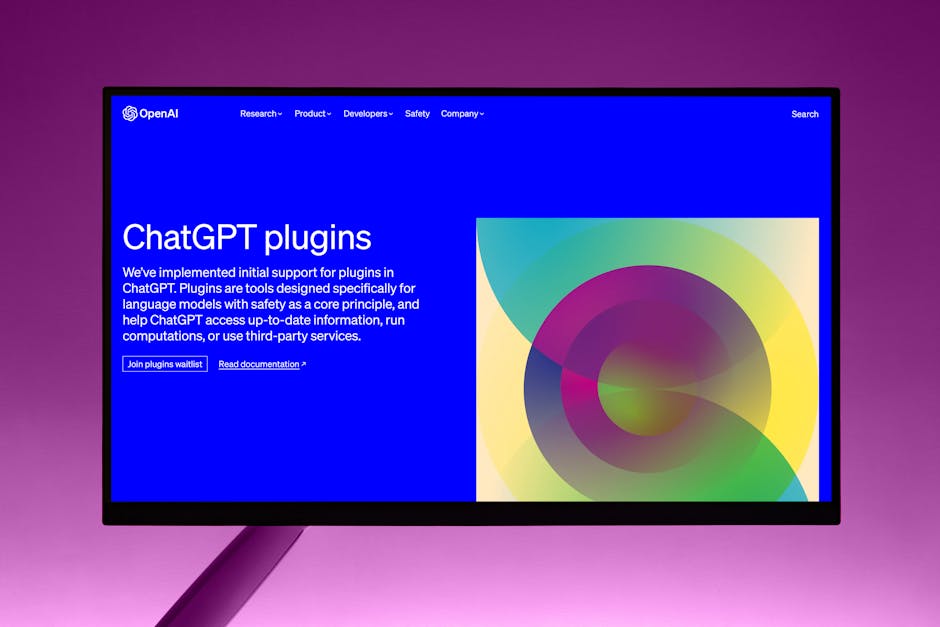
While implementing infinite scroll functionality using React and MongoDB, I encountered an issue where data was duplicating at pagination boundaries. This article explores the cause of this problem, which stems from MongoDB's sorting behavior, and presents a concrete solution using the _id field.
Developers working with React and MongoDB applications.
Those implementing or planning to implement infinite scroll/pagination.
Anyone interested in understanding database sorting behaviors.
MongoDB's sorting behavior can lead to inconsistent ordering of documents with identical sort values. This causes key duplication warnings in React infinite scroll implementations. The solution involves adding the _id field as a secondary sort key.
- React [website] - MongoDB [website] - MongoDB [website] Driver [website] - TypeScript [website] - [website] [website] Enter fullscreen mode Exit fullscreen mode.
When querying a MongoDB Collection with a large dataset using filters, duplicate data appeared in the infinite scroll display. Specifically, the last item from the first fetch overlapped with the first item from the second fetch.
Warning: Encountered two children with the same key, `.$xxxxxxxxxxxx`. Keys should be unique so that components maintain their identity across updates. Non-unique keys may cause children to be duplicated and/or omitted — the behavior is unsupported and could change in a future version. Enter fullscreen mode Exit fullscreen mode.
MongoDB query includes filter conditions.
// Backend: MongoDB query const sortObject = { [ sortBy ]: order === " asc " ? 1 : - 1 }; const items = await Collection . find ( query ) . sort ( sortObject ) . skip ( skip ) . limit ( limit ) . lean () . exec (); // Frontend: React component const loadMore = async ( page : number ) => { const response = await fetchItems ( page ); setState (( prev ) => ({ items : [... prev . items , ... response . items ], page , })); }; Enter fullscreen mode Exit fullscreen mode.
MongoDB Sorting Behavior Characteristics.
Documents with identical sort values don't maintain consistent ordering When combined with pagination ( skip and limit ), the same document might appear on different pages This results in React displaying items with duplicate keys.
Reference: MongoDB Documentation: sort().
const sortObject = { [ sortBy ]: order === " asc " ? 1 : - 1 , _id : 1 // Add _id as a secondary sort key }; const items = await Collection . find ( query ) . sort ( sortObject ) . skip ( skip ) . limit ( limit ) . lean () . exec (); Enter fullscreen mode Exit fullscreen mode.
The _id field in MongoDB guarantees uniqueness When primary sort keys have equal values, _id ordering ensures consistent results This prevents pagination duplication.
By default, the order of documents with identical sort values is indeterminate.
This behavior becomes more pronounced in distributed systems and sharding scenarios.
Stable sorting requires including a unique value in the sort keys.
When implementing infinite scroll with MongoDB, sorting behavior can cause issues in these scenarios:
Sort fields contain duplicate values Pagination boundaries in large datasets Multiple filter condition combinations.
Utilizing the _id field as a secondary sort key enables stable sort results and clean infinite scroll implementation.
There are times when a new feature containing sorting is introduced. Obviously, we want to verify that the implemented sorting works correctly. Assert......
The Azure AI Agent Service is Microsoft’s enterprise-grade implementation of AI agents. It empowers developers to build, deploy, and scale sophisticat......
At the ASF's flagship Community Over Code North America conference in October 2024, keynote speakers underscored the vital role of open-source communi......
Market Impact Analysis
Market Growth Trend
2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|
7.5% | 9.0% | 9.4% | 10.5% | 11.0% | 11.4% | 11.5% |
Quarterly Growth Rate
Q1 2024 | Q2 2024 | Q3 2024 | Q4 2024 |
---|---|---|---|
10.8% | 11.1% | 11.3% | 11.5% |
Market Segments and Growth Drivers
Segment | Market Share | Growth Rate |
---|---|---|
Enterprise Software | 38% | 10.8% |
Cloud Services | 31% | 17.5% |
Developer Tools | 14% | 9.3% |
Security Software | 12% | 13.2% |
Other Software | 5% | 7.5% |
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity:
Competitive Landscape Analysis
Company | Market Share |
---|---|
Microsoft | 22.6% |
Oracle | 14.8% |
SAP | 12.5% |
Salesforce | 9.7% |
Adobe | 8.3% |
Future Outlook and Predictions
The Install Arch Swapfile landscape is evolving rapidly, driven by technological advancements, changing threat vectors, and shifting business requirements. Based on current trends and expert analyses, we can anticipate several significant developments across different time horizons:
Year-by-Year Technology Evolution
Based on current trajectory and expert analyses, we can project the following development timeline:
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity, influencing adoption timelines and investment priorities:
Innovation Trigger
- Generative AI for specialized domains
- Blockchain for supply chain verification
Peak of Inflated Expectations
- Digital twins for business processes
- Quantum-resistant cryptography
Trough of Disillusionment
- Consumer AR/VR applications
- General-purpose blockchain
Slope of Enlightenment
- AI-driven analytics
- Edge computing
Plateau of Productivity
- Cloud infrastructure
- Mobile applications
Technology Evolution Timeline
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
Expert Perspectives
Leading experts in the software dev sector provide diverse perspectives on how the landscape will evolve over the coming years:
"Technology transformation will continue to accelerate, creating both challenges and opportunities."
— Industry Expert
"Organizations must balance innovation with practical implementation to achieve meaningful results."
— Technology Analyst
"The most successful adopters will focus on business outcomes rather than technology for its own sake."
— Research Director
Areas of Expert Consensus
- Acceleration of Innovation: The pace of technological evolution will continue to increase
- Practical Integration: Focus will shift from proof-of-concept to operational deployment
- Human-Technology Partnership: Most effective implementations will optimize human-machine collaboration
- Regulatory Influence: Regulatory frameworks will increasingly shape technology development
Short-Term Outlook (1-2 Years)
In the immediate future, organizations will focus on implementing and optimizing currently available technologies to address pressing software dev challenges:
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
These developments will be characterized by incremental improvements to existing frameworks rather than revolutionary changes, with emphasis on practical deployment and measurable outcomes.
Mid-Term Outlook (3-5 Years)
As technologies mature and organizations adapt, more substantial transformations will emerge in how security is approached and implemented:
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
This period will see significant changes in security architecture and operational models, with increasing automation and integration between previously siloed security functions. Organizations will shift from reactive to proactive security postures.
Long-Term Outlook (5+ Years)
Looking further ahead, more fundamental shifts will reshape how cybersecurity is conceptualized and implemented across digital ecosystems:
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
These long-term developments will likely require significant technical breakthroughs, new regulatory frameworks, and evolution in how organizations approach security as a fundamental business function rather than a technical discipline.
Key Risk Factors and Uncertainties
Several critical factors could significantly impact the trajectory of software dev evolution:
Organizations should monitor these factors closely and develop contingency strategies to mitigate potential negative impacts on technology implementation timelines.
Alternative Future Scenarios
The evolution of technology can follow different paths depending on various factors including regulatory developments, investment trends, technological breakthroughs, and market adoption. We analyze three potential scenarios:
Optimistic Scenario
Rapid adoption of advanced technologies with significant business impact
Key Drivers: Supportive regulatory environment, significant research breakthroughs, strong market incentives, and rapid user adoption.
Probability: 25-30%
Base Case Scenario
Measured implementation with incremental improvements
Key Drivers: Balanced regulatory approach, steady technological progress, and selective implementation based on clear ROI.
Probability: 50-60%
Conservative Scenario
Technical and organizational barriers limiting effective adoption
Key Drivers: Restrictive regulations, technical limitations, implementation challenges, and risk-averse organizational cultures.
Probability: 15-20%
Scenario Comparison Matrix
Factor | Optimistic | Base Case | Conservative |
---|---|---|---|
Implementation Timeline | Accelerated | Steady | Delayed |
Market Adoption | Widespread | Selective | Limited |
Technology Evolution | Rapid | Progressive | Incremental |
Regulatory Environment | Supportive | Balanced | Restrictive |
Business Impact | Transformative | Significant | Modest |
Transformational Impact
Technology becoming increasingly embedded in all aspects of business operations. This evolution will necessitate significant changes in organizational structures, talent development, and strategic planning processes.
The convergence of multiple technological trends—including artificial intelligence, quantum computing, and ubiquitous connectivity—will create both unprecedented security challenges and innovative defensive capabilities.
Implementation Challenges
Technical complexity and organizational readiness remain key challenges. Organizations will need to develop comprehensive change management strategies to successfully navigate these transitions.
Regulatory uncertainty, particularly around emerging technologies like AI in security applications, will require flexible security architectures that can adapt to evolving compliance requirements.
Key Innovations to Watch
Artificial intelligence, distributed systems, and automation technologies leading innovation. Organizations should monitor these developments closely to maintain competitive advantages and effective security postures.
Strategic investments in research partnerships, technology pilots, and talent development will position forward-thinking organizations to leverage these innovations early in their development cycle.
Technical Glossary
Key technical terms and definitions to help understand the technologies discussed in this article.
Understanding the following technical concepts is essential for grasping the full implications of the security threats and defensive measures discussed in this article. These definitions provide context for both technical and non-technical readers.