Read Response Body in JAX-RS Client from a POST Request - Related to kafka, body, a, options, read
Kafka Message Acknowledgement Options
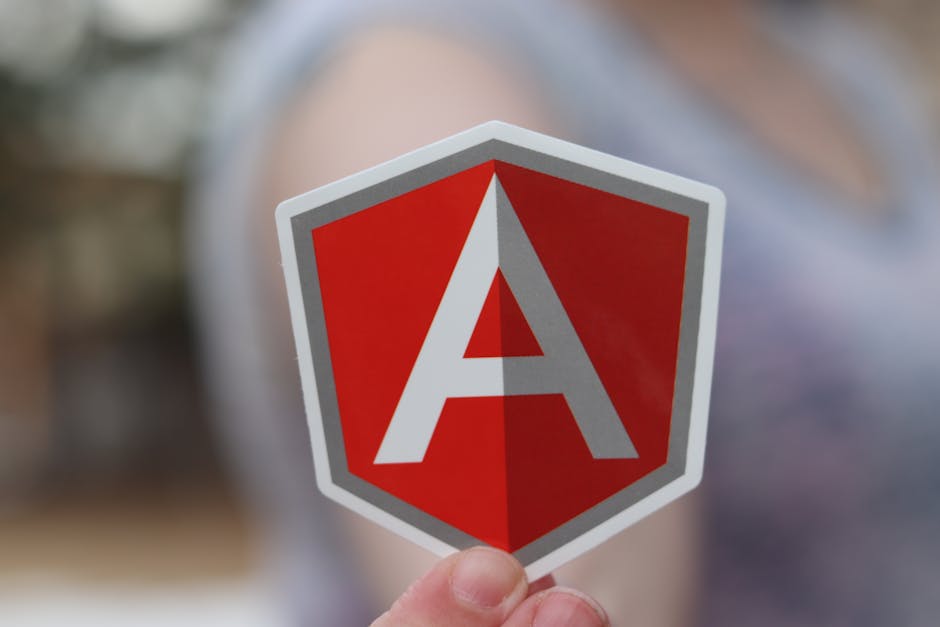
Apache Kafka is a distributed messaging system widely used for building real-time data pipelines and streaming applications. To ensure reliable message delivery, Kafka provides various message acknowledgment options for both producers and consumers. These options help balance performance, durability, and fault tolerance based on application needs. Producers can control how messages are acknowledged by brokers, while consumers manage message processing guarantees. Understanding these acknowledgment mechanisms is crucial for designing efficient Kafka-based systems. Let us delve into understanding Kafka message acknowledgment options and explore how they impact reliability, message ordering, and system resilience through practical Java examples.
Scalability: Kafka supports horizontal scaling by adding more brokers to the cluster.
Fault Tolerance: Data replication ensures message durability even if a broker fails.
High Throughput: Kafka processes millions of messages per second with low latency.
Durability: Messages are stored persistently, ensuring reliability.
Flexibility: Kafka integrates seamlessly with big data and cloud-based platforms.
Kafka producers can configure acknowledgments using the acks parameter. This setting determines when a producer considers a message as successfully sent.
acks=0 : The producer does not wait for acknowledgment from the broker. The message is sent asynchronously without waiting for confirmation, making it the fastest option but with a higher risk of data loss if the broker fails.
: The producer does not wait for acknowledgment from the broker. The message is sent asynchronously without waiting for confirmation, making it the fastest option but with a higher risk of data loss if the broker fails. acks=1 : The producer waits for acknowledgment from the leader only. If the leader broker receives the message and confirms it, the producer considers it successful. However, if the leader fails before replicating the data, the message might be lost.
: The producer waits for acknowledgment from the leader only. If the leader broker receives the message and confirms it, the producer considers it successful. However, if the leader fails before replicating the data, the message might be lost. acks=all (or -1 ): The producer waits for acknowledgment from all in-sync replicas (ISR). This ensures the highest level of durability, as messages are not considered sent until all replicas have confirmed receipt. This option provides strong consistency but may introduce latency.
[website] Choosing the Right Acknowledgment Strategy.
Choosing the right acknowledgment level depends on the application requirements:
For high throughput with low latency and tolerance for message loss, use acks=0 .
. For a balance between reliability and performance, use acks=1 .
. For the highest durability and no data loss, use acks=all .
[website] Java Code Example for Kafka Producer.
import [website]*; import [website]; public class KafkaProducerExample { public static void main(String[] args) { String topicName = "test-topic"; Properties props = new Properties(); [website]"bootstrap.servers", "localhost:9092"); [website]"key.serializer", "[website]"); [website]"value.serializer", "[website]"); // Setting acknowledgment level to 'all' [website]"acks", "all"); KafkaProducer producer = new KafkaProducer<>(props); for (int i = 1; i <= 5; i++) { ProducerRecord record = new ProducerRecord<>(topicName, "Key" + i, "Message" + i); [website], (metadata, exception) -> { if (exception == null) { [website]"Sent message to topic: " + [website] + " | Partition: " + metadata.partition() + " | Offset: " + [website]; } else { [website]"Error while producing: " + exception.getMessage()); } }); } [website]; } }.
This Java program demonstrates a simple Kafka producer that sends messages to a Kafka topic named “test-topic.” It first sets up a Properties object with essential Kafka configurations, including the bootstrap server ( localhost:9092 ), key and value serializers ( StringSerializer ), and acknowledgment level ( acks=all ) to ensure message durability. A KafkaProducer instance is created using these properties, and a loop iterates five times to send messages with keys ( Key1 to Key5 ) and values ( Message1 to Message5 ). Each message is wrapped in a ProducerRecord and sent asynchronously using [website] , which includes a callback to log metadata (topic, partition, and offset) on successful delivery or print an error message if the send fails. Finally, the producer is closed to release resources.
Sent message to topic: test-topic | Partition: 0 | Offset: 10 Sent message to topic: test-topic | Partition: 1 | Offset: 8 Sent message to topic: test-topic | Partition: 2 | Offset: 15 Sent message to topic: test-topic | Partition: 0 | Offset: 11 Sent message to topic: test-topic | Partition: 1 | Offset: 9.
[website] Challenges in Producer Acknowledgments.
Latency vs. Reliability: Higher acknowledgment levels increase reliability but add latency.
Message Duplication: Retries may lead to duplicate messages.
Leader Failures: Messages may be lost if the leader crashes before replication.
Kafka consumers use manual or automatic acknowledgments to ensure message processing reliability. Consumers track their read positions using offsets, and acknowledgment determines when the offset is committed.
[website] : The consumer automatically commits offsets at a regular interval. This setting reduces overhead but risks message loss in case of failure, as messages are considered processed even before actual consumption.
: The consumer automatically commits offsets at a regular interval. This setting reduces overhead but risks message loss in case of failure, as messages are considered processed even before actual consumption. [website] : The consumer manually commits offsets after processing messages. This approach ensures reliability by committing offsets only when processing is complete, preventing message loss but requiring additional management.
Keep in mind that when [website] , Kafka offers two methods for committing offsets:
commitSync() : Commits offsets synchronously, ensuring they are written to Kafka before proceeding. This approach guarantees message acknowledgment but may introduce latency.
: Commits offsets synchronously, ensuring they are written to Kafka before proceeding. This approach guarantees message acknowledgment but may introduce latency. commitAsync() : Commits offsets asynchronously, improving performance but risking data loss if the application crashes before completion.
[website] Choosing the Right Acknowledgment Strategy for Consumers.
Choosing the right acknowledgment strategy depends on your application’s needs:
For simple processing where some message loss is acceptable, use [website] .
. For critical applications requiring precise message handling, use [website] with commitSync() .
with . For high-performance scenarios with reduced acknowledgment overhead, use commitAsync() , but handle potential duplicate processing.
[website] Java Code Example for Kafka Consumer.
Consumed message: Message1 | Offset: 10 Consumed message: Message2 | Offset: 8 Consumed message: Message3 | Offset: 15 Consumed message: Message4 | Offset: 11 Consumed message: Message5 | Offset: 9.
One quality every engineering manager should have? Empathy.
Ryan talks with senior engineering manager Caitlin Weaver about how he......
Pinecone unveiled Tuesday the next generation version of its serverless architecture, which the organization says is designed to advanced support a wide var......
Around 30,000 websites and applications are hacked every day*, and the developer is often to blame.
The vast majority of breaches occur due to miscon......
Read Response Body in JAX-RS Client from a POST Request
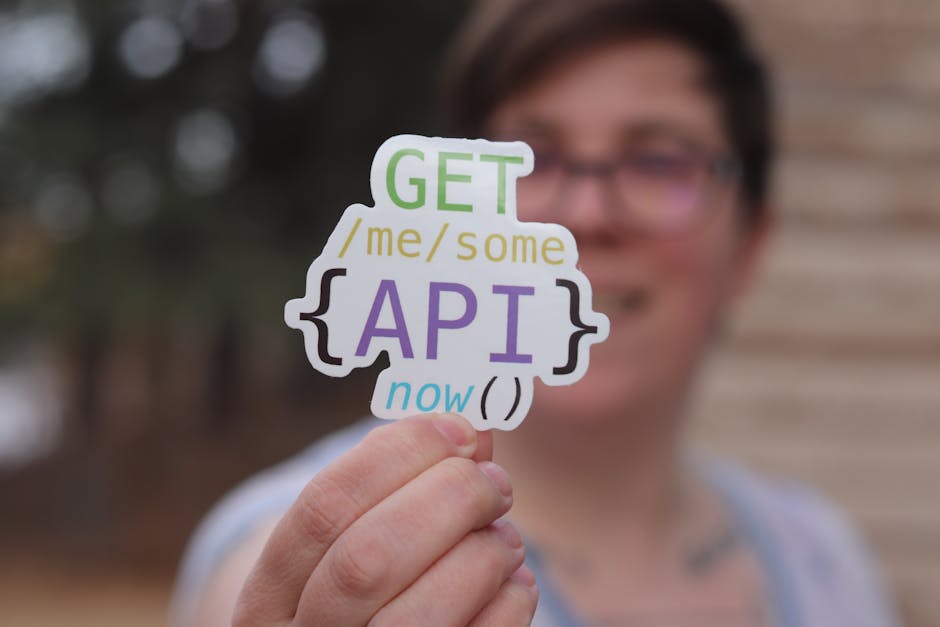
JAX-RS (Jakarta API for RESTful Web Services) is a widely used framework for building RESTful web services in Java. It provides a client API that allows us to interact with RESTful services. When making a POST request using the JAX-RS client, we often need to read the response body returned by the server. This article will guide you through the process of reading the response body from a POST request using the JAX-RS client.
To use JAX-RS client attributes, add the following dependencies to your [website] . We will use Jersey, the reference implementation of JAX-RS.
[website] jersey-client [website] [website] jersey-media-json-binding [website].
2. Creating a Simple REST API (for Testing).
Before we create the client, let’s define a simple JAX-RS server to test our POST request. Assume we are sending a POST request to /api/people with user details in JSON format.
@Path("/customers") public class UserResource { @POST @Consumes(MediaType.APPLICATION_JSON) @Produces(MediaType.APPLICATION_JSON) public Response createUser(User user) { [website]; // Simulate ID assignment return [website]; } }.
public class User { private int id; private String name; private String email; public User() { } public User(String name, String email) { [website] = name; [website] = email; } public int getId() { return id; } public void setId(int id) { [website] = id; } public String getName() { return name; } public void setName(String name) { [website] = name; } public String getEmail() { return email; } public void setEmail(String email) { [website] = email; } }.
This API accepts a POST request with a User object in JSON format, assigns it an ID, and returns the updated user as a response.
Now, let’s create a JAX-RS client to send a POST request and read the response body.
public class JaxrsClientExample { public static void main(String[] args) { // Create JAX-RS Client Client client = ClientBuilder.newClient(); // Define the target URL WebTarget target = [website]"[website]:8080/api/consumers"); // Create the user request payload User user = new User("John Fish", "[website]"); // Send POST request Response response = target.request(MediaType.APPLICATION_JSON) .post([website], MediaType.APPLICATION_JSON)); // Read response body as String String jsonResponse = response.readEntity([website]; [website]"Response as String: " + jsonResponse); // Read response body as a User object User createdUser = response.readEntity([website]; [website]"Response as User Object: " + [website] + ", " + createdUser.getName()); [website]; } }.
To begin, we need to create an instance of the JAX-RS client, which acts as the foundation for making HTTP requests. This is achieved using Client client = ClientBuilder.newClient(); , which initializes a new JAX-RS client instance. Once the client is ready, we define the target API endpoint using WebTarget target = [website]"[website]:8080/api/consumers"); . This establishes the base URL for our requests, allowing us to interact with the specified RESTful service.
When sending a POST request, we use post([website], MediaType.APPLICATION_JSON)) , which takes a User object and converts it into a JSON payload. This ensures that the data is formatted correctly before being transmitted to the server.
After making the request, reading the response body is crucial for handling the server’s response. The simplest way to retrieve the response is as a raw JSON string using response.readEntity([website] . This is useful when we want to process or log the raw response manually.
However, in many cases, we want to work directly with a Java object. Using response.readEntity([website] , we can automatically convert the JSON response into a User object, leveraging Jackson’s JSON binding capabilities. This simplifies data handling by allowing us to interact with the response as a strongly typed Java object instead of parsing JSON manually.
Finally, to ensure efficient resource management, we close the client using [website]; , which releases any underlying resources and prevents potential memory leaks.
It is essential to check the HTTP status before reading the response body.
if (response.getStatus() == 200) { User createdUser = response.readEntity([website]; [website]"User Created: " + createdUser.getName()); } else { [website]"Error: " + response.getStatus() + " - " + response.readEntity([website]; }.
Start the JAX-RS server (full source code for the JAX-RS server is available from the download section), run the JAX-RS client, and you should see an output similar to the following.
Response as String: {"email":"[website]","id":1001,"name":"John Fish"} Response as User Object: 1001, John Fish.
This article demonstrated how to use the JAX-RS client API to send a POST request and read the response body in different formats. We covered setting up dependencies, creating a simple REST API, implementing the client, and handling errors. This approach allows seamless integration with RESTful services while leveraging the power of JAX-RS.
This article explored how to read the response body using a JAX-RS client.
Kubectl is the command-line interface for interacting with Kubernetes clusters. It allows you to deploy applications, insp......
Microsoft annonce que Skype fermera définitivement le 5 mai prochain. L'éditeur avait racheté la solution de communication en 2011 pour la modique som......
If you’re heading to KubeCon Europe in London this April, chances are you’re not paying to sample the food. You’re going for the sessions and specific......
Subroutines: Interview Problem Survey
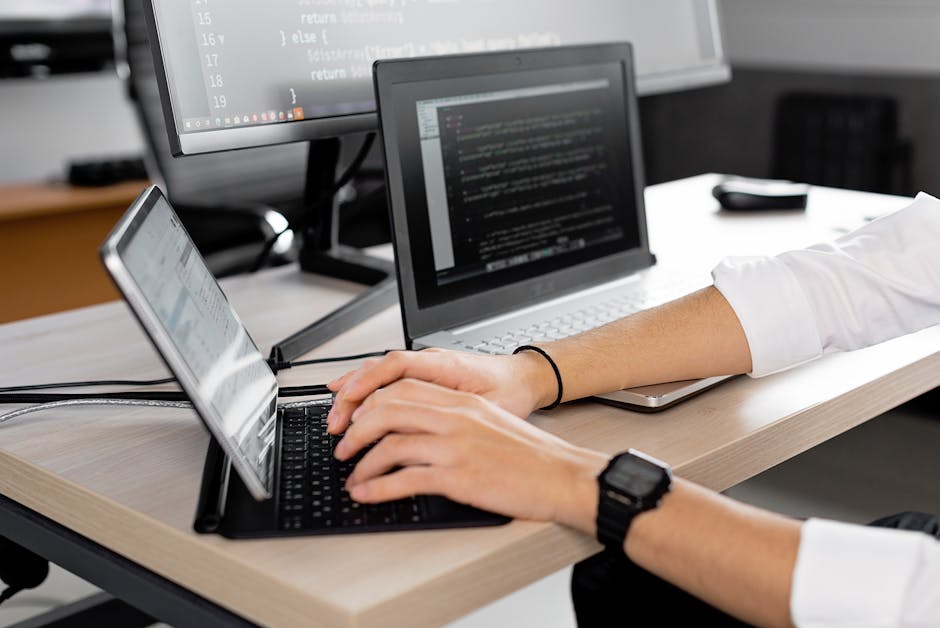
Lets say we have an algorithm which performs a check if a string is a palindrome.
bool isPalindrome(string s) { for(int i = 0; i < [website]; i++) { if([website] != [website] { return false; } } return true; } Enter fullscreen mode Exit fullscreen mode.
This type of solution can be a subroutine to many questions; which may require pre-processing before calling isPalindrome() . An example may be removing spaces and alphanumeric characters.
bool cleanString(string s) { string cleanedstr = ""; for(char c : s) { if(isalnum(c)) { cleanedstr += tolower(c); } } return cleanedstr; } Enter fullscreen mode Exit fullscreen mode.
To superior use the isPalindrome as a subroutine we can add additional parameters. This can help answering questions like leetcode 680; where at most one character can be deleted.
bool isPalindrome(string s, int i, int j) { for(;iand the parent function handles the case where we may have up to one deleted [website] atMostOneMissingPalendrome() { int i = 0; int j = [website] -1; for(int i = 0, j = [website]; iNow a more common scenario during an interview is having to use depth-first search or breadth first search. One question where this appears is connected-components or connected islands style questions; where you are given a matrix and asked to find the number of islands or the biggest island where a '1' denotes sand presence and '0' denotes water.example instance;111000 100011 001010 Enter fullscreen mode Exit fullscreen modeexample solution;4 Enter fullscreen mode Exit fullscreen modeexample code;int depth_first_search(vector> matrix, int i, int j) { if([website] == 0) { return 0; } int total = 1; if(i+1 < [website] total += depth_first_search(matrix, i+1, j); if(i-1 >= 0) total += depth_first_search(matrix, i-1, j); if(j+1 < [website] total += depth_first_search(matrix, i, j+1); if(j-1 >= 0) total += depth_first_search(matrix, i, j-1); return total; } int find_largest_island_size(vector> matrix) { int max_island = 0; for(int i = 0; i < [website]; i++) { for(int j = 0; j < [website]; j++) { max_island = max(max_island, depth_first_search(matrix, i, j)); } } return max_island } Enter fullscreen mode Exit fullscreen modeNow we may have scenarios where instead of the classic up, down, left, right search options we have more complex operators such as in leetcode 282. Where we are given a String of numbers and a target number and can introduce binary operators which result in the expression resolving to the target number. The goal is to find all such expressionsexample instance;"332" 8 Enter fullscreen mode Exit fullscreen modeexample solution;3+3+2 Enter fullscreen mode Exit fullscreen modeexample code;
and the parent function handles the case where we may have up to one deleted character.
bool atMostOneMissingPalendrome() { int i = 0; int j = [website] -1; for(int i = 0, j = [website]; iNow a more common scenario during an interview is having to use depth-first search or breadth first search. One question where this appears is connected-components or connected islands style questions; where you are given a matrix and asked to find the number of islands or the biggest island where a '1' denotes sand presence and '0' denotes water.example instance;111000 100011 001010 Enter fullscreen mode Exit fullscreen modeexample solution;4 Enter fullscreen mode Exit fullscreen modeexample code;int depth_first_search(vector> matrix, int i, int j) { if([website] == 0) { return 0; } int total = 1; if(i+1 < [website] total += depth_first_search(matrix, i+1, j); if(i-1 >= 0) total += depth_first_search(matrix, i-1, j); if(j+1 < [website] total += depth_first_search(matrix, i, j+1); if(j-1 >= 0) total += depth_first_search(matrix, i, j-1); return total; } int find_largest_island_size(vector> matrix) { int max_island = 0; for(int i = 0; i < [website]; i++) { for(int j = 0; j < [website]; j++) { max_island = max(max_island, depth_first_search(matrix, i, j)); } } return max_island } Enter fullscreen mode Exit fullscreen modeNow we may have scenarios where instead of the classic up, down, left, right search options we have more complex operators such as in leetcode 282. Where we are given a String of numbers and a target number and can introduce binary operators which result in the expression resolving to the target number. The goal is to find all such expressionsexample instance;"332" 8 Enter fullscreen mode Exit fullscreen modeexample solution;3+3+2 Enter fullscreen mode Exit fullscreen modeexample code;
Now a more common scenario during an interview is having to use depth-first search or breadth first search. One question where this appears is connected-components or connected islands style questions; where you are given a matrix and asked to find the number of islands or the biggest island where a '1' denotes sand presence and '0' denotes water.
111000 100011 001010 Enter fullscreen mode Exit fullscreen mode.
4 Enter fullscreen mode Exit fullscreen mode.
int depth_first_search(vector> matrix, int i, int j) { if([website] == 0) { return 0; } int total = 1; if(i+1 < [website] total += depth_first_search(matrix, i+1, j); if(i-1 >= 0) total += depth_first_search(matrix, i-1, j); if(j+1 < [website] total += depth_first_search(matrix, i, j+1); if(j-1 >= 0) total += depth_first_search(matrix, i, j-1); return total; } int find_largest_island_size(vector> matrix) { int max_island = 0; for(int i = 0; i < [website]; i++) { for(int j = 0; j < [website]; j++) { max_island = max(max_island, depth_first_search(matrix, i, j)); } } return max_island } Enter fullscreen mode Exit fullscreen mode.
Now we may have scenarios where instead of the classic up, down, left, right search options we have more complex operators such as in leetcode 282. Where we are given a String of numbers and a target number and can introduce binary operators which result in the expression resolving to the target number. The goal is to find all such expressions.
"332" 8 Enter fullscreen mode Exit fullscreen mode.
3+3+2 Enter fullscreen mode Exit fullscreen mode.
Animations can elevate the user experience in mobile apps, making them more engaging and intuitive. One of the best libraries for adding smooth, light......
It was only a month ago that DeepSeek disrupted the AI world with its brilliant use of optimization and leveraging of the NVIDIA GPU's the team had to......
The tables in a database form the foundations of data-driven applications. Laboring with a schema that’s a haphazard muddle of confusing names and dat......
Market Impact Analysis
Market Growth Trend
2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|
7.5% | 9.0% | 9.4% | 10.5% | 11.0% | 11.4% | 11.5% |
Quarterly Growth Rate
Q1 2024 | Q2 2024 | Q3 2024 | Q4 2024 |
---|---|---|---|
10.8% | 11.1% | 11.3% | 11.5% |
Market Segments and Growth Drivers
Segment | Market Share | Growth Rate |
---|---|---|
Enterprise Software | 38% | 10.8% |
Cloud Services | 31% | 17.5% |
Developer Tools | 14% | 9.3% |
Security Software | 12% | 13.2% |
Other Software | 5% | 7.5% |
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity:
Competitive Landscape Analysis
Company | Market Share |
---|---|
Microsoft | 22.6% |
Oracle | 14.8% |
SAP | 12.5% |
Salesforce | 9.7% |
Adobe | 8.3% |
Future Outlook and Predictions
The Kafka Message Acknowledgement landscape is evolving rapidly, driven by technological advancements, changing threat vectors, and shifting business requirements. Based on current trends and expert analyses, we can anticipate several significant developments across different time horizons:
Year-by-Year Technology Evolution
Based on current trajectory and expert analyses, we can project the following development timeline:
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity, influencing adoption timelines and investment priorities:
Innovation Trigger
- Generative AI for specialized domains
- Blockchain for supply chain verification
Peak of Inflated Expectations
- Digital twins for business processes
- Quantum-resistant cryptography
Trough of Disillusionment
- Consumer AR/VR applications
- General-purpose blockchain
Slope of Enlightenment
- AI-driven analytics
- Edge computing
Plateau of Productivity
- Cloud infrastructure
- Mobile applications
Technology Evolution Timeline
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
Expert Perspectives
Leading experts in the software dev sector provide diverse perspectives on how the landscape will evolve over the coming years:
"Technology transformation will continue to accelerate, creating both challenges and opportunities."
— Industry Expert
"Organizations must balance innovation with practical implementation to achieve meaningful results."
— Technology Analyst
"The most successful adopters will focus on business outcomes rather than technology for its own sake."
— Research Director
Areas of Expert Consensus
- Acceleration of Innovation: The pace of technological evolution will continue to increase
- Practical Integration: Focus will shift from proof-of-concept to operational deployment
- Human-Technology Partnership: Most effective implementations will optimize human-machine collaboration
- Regulatory Influence: Regulatory frameworks will increasingly shape technology development
Short-Term Outlook (1-2 Years)
In the immediate future, organizations will focus on implementing and optimizing currently available technologies to address pressing software dev challenges:
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
These developments will be characterized by incremental improvements to existing frameworks rather than revolutionary changes, with emphasis on practical deployment and measurable outcomes.
Mid-Term Outlook (3-5 Years)
As technologies mature and organizations adapt, more substantial transformations will emerge in how security is approached and implemented:
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
This period will see significant changes in security architecture and operational models, with increasing automation and integration between previously siloed security functions. Organizations will shift from reactive to proactive security postures.
Long-Term Outlook (5+ Years)
Looking further ahead, more fundamental shifts will reshape how cybersecurity is conceptualized and implemented across digital ecosystems:
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
These long-term developments will likely require significant technical breakthroughs, new regulatory frameworks, and evolution in how organizations approach security as a fundamental business function rather than a technical discipline.
Key Risk Factors and Uncertainties
Several critical factors could significantly impact the trajectory of software dev evolution:
Organizations should monitor these factors closely and develop contingency strategies to mitigate potential negative impacts on technology implementation timelines.
Alternative Future Scenarios
The evolution of technology can follow different paths depending on various factors including regulatory developments, investment trends, technological breakthroughs, and market adoption. We analyze three potential scenarios:
Optimistic Scenario
Rapid adoption of advanced technologies with significant business impact
Key Drivers: Supportive regulatory environment, significant research breakthroughs, strong market incentives, and rapid user adoption.
Probability: 25-30%
Base Case Scenario
Measured implementation with incremental improvements
Key Drivers: Balanced regulatory approach, steady technological progress, and selective implementation based on clear ROI.
Probability: 50-60%
Conservative Scenario
Technical and organizational barriers limiting effective adoption
Key Drivers: Restrictive regulations, technical limitations, implementation challenges, and risk-averse organizational cultures.
Probability: 15-20%
Scenario Comparison Matrix
Factor | Optimistic | Base Case | Conservative |
---|---|---|---|
Implementation Timeline | Accelerated | Steady | Delayed |
Market Adoption | Widespread | Selective | Limited |
Technology Evolution | Rapid | Progressive | Incremental |
Regulatory Environment | Supportive | Balanced | Restrictive |
Business Impact | Transformative | Significant | Modest |
Transformational Impact
Technology becoming increasingly embedded in all aspects of business operations. This evolution will necessitate significant changes in organizational structures, talent development, and strategic planning processes.
The convergence of multiple technological trends—including artificial intelligence, quantum computing, and ubiquitous connectivity—will create both unprecedented security challenges and innovative defensive capabilities.
Implementation Challenges
Technical complexity and organizational readiness remain key challenges. Organizations will need to develop comprehensive change management strategies to successfully navigate these transitions.
Regulatory uncertainty, particularly around emerging technologies like AI in security applications, will require flexible security architectures that can adapt to evolving compliance requirements.
Key Innovations to Watch
Artificial intelligence, distributed systems, and automation technologies leading innovation. Organizations should monitor these developments closely to maintain competitive advantages and effective security postures.
Strategic investments in research partnerships, technology pilots, and talent development will position forward-thinking organizations to leverage these innovations early in their development cycle.
Technical Glossary
Key technical terms and definitions to help understand the technologies discussed in this article.
Understanding the following technical concepts is essential for grasping the full implications of the security threats and defensive measures discussed in this article. These definitions provide context for both technical and non-technical readers.