Jio Platforms to Build Open Telecom AI Platform with AMD, Cisco and Nokia - Related to science:, science, agent, google, open
Data Science: From School to Work, Part II
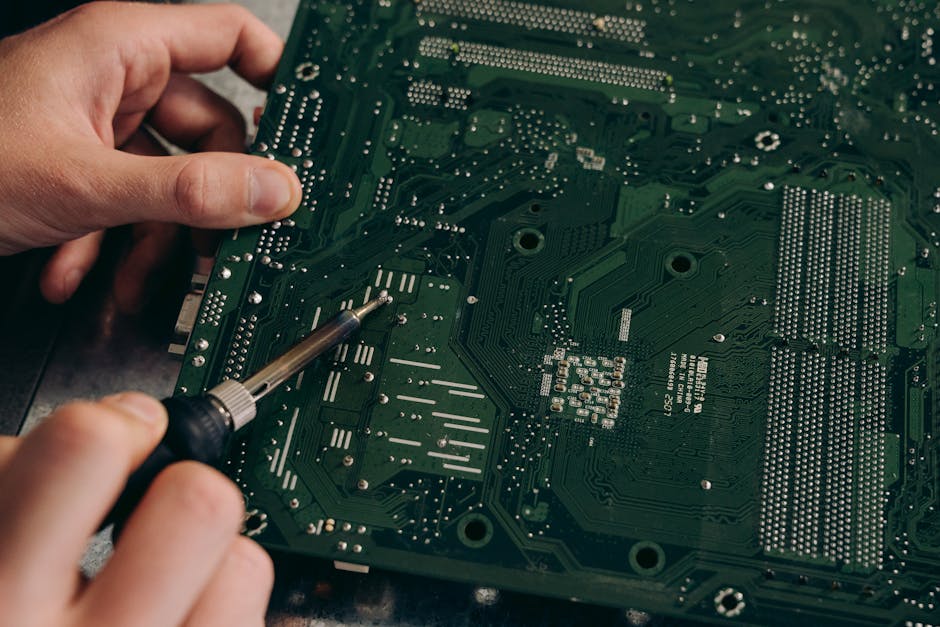
In my previous article, I highlighted the importance of effective project management in Python development. Now, let’s shift our focus to the code itself and explore how to write clean, maintainable code — an essential practice in professional and collaborative environments.
Readability & Maintainability: Well-structured code is easier to read. Understand, and modify. Other developers — or even your future self — can quickly grasp the logic without struggling to decipher messy code.
Well-structured code is easier to read, understand. And modify. Other developers — or even your future self — can quickly grasp the logic without struggling to decipher messy code. Debugging & Troubleshooting: Organized code with clear variable names and structured functions makes it easier to identify and fix bugs efficiently.
Organized code with clear variable names and. Structured functions makes it easier to identify and fix bugs efficiently. Scalability & Reusability: Modular, well-organized code can be reused across different projects, allowing for seamless scaling without disrupting existing functionality.
So, as you work on your next Python project, remember:
Python is one of the most popular and versatile Programming languages. Appreciated for its simplicity, comprehensibility and large community. Whether web development, data analysis, artificial intelligence or automation of tasks — Python offers powerful and flexible tools that are suitable for a wide range of areas.
However, the efficiency and. Maintainability of a Python project depends heavily on the practices used by the developers. Poor structuring of the code, a lack of conventions or even a lack of documentation can quickly turn a promising project into a maintenance and. Development-intensive puzzle. It is precisely this point that makes the difference between student code and. Professional code.
This article is intended to present the most crucial best practices for writing high-quality Python code. By following these recommendations, developers can create scripts and applications that are not only functional, but also readable, performant and easily maintainable by third parties.
Adopting these best practices right from the start of a project not only ensures more effective collaboration within teams. But also prepares your code to evolve with future needs. Whether you’re a beginner or an experienced developer, this guide is designed to support you in all your Python developments.
Good code structuring in Python is essential. There are two main project layouts: flat layout and src layout.
The flat layout places the source code directly in the project root without an additional folder. This approach simplifies the structure and is well-suited for small scripts, quick prototypes, and projects that do not require complex packaging. However, it may lead to unintended import issues when running tests or scripts.
📂 my_project/ ├── 📂 my_project/ # Directly in the root │ ├── 🐍 │ ├── 🐍 # Main entry point (if needed) │ ├── 🐍 # Example module │ └── 🐍 ├── 📂 tests/ # Unit tests │ ├── 🐍 │ ├── 🐍 │ └── ... ├── 📄 . gitignore # Git ignored files ├── 📄 # Project configuration (Poetry, setuptools) ├── 📄 # UV file ├── 📄 # Main project documentation ├── 📄 LICENSE # Project license ├── 📄 Makefile # Automates common tasks ├── 📄 DockerFile # Automates common tasks ├── 📂 . github/ # GitHub Actions workflows (CI/CD) │ ├── 📂 actions/ │ └── 📂 workflows/.
On the other hand, the src layout (src is the contraction of source) organizes the source code inside a dedicated src/ directory, preventing accidental imports from the working directory and. Ensuring a clear separation between source files and other project components like tests or configuration files. This layout is ideal for large projects, libraries, and production-ready applications as it enforces proper package installation and avoids import conflicts.
📂 my-project/ ├── 📂 src/ # Main source code │ ├── 📂 my_project/ # Main package │ │ ├── 🐍 # Makes the folder a package │ │ ├── 🐍 # Main entry point (if needed) │ │ ├── 🐍 # Example module │ │ └── ... │ │ ├── 📂 utils/ # Utility functions │ │ │ ├── 🐍 │ │ │ ├── 🐍 # data functions │ │ │ ├── 🐍 # Input/output functions │ │ │ └── ... ├── 📂 tests/ # Unit tests │ ├── 🐍 │ ├── 🐍 │ ├── 🐍 # Pytest configurations │ └── ... ├── 📂 docs/ # Documentation │ ├── 📄 │ ├── 📄 │ ├── 📄 │ └── ... ├── 📂 notebooks/ # Jupyter Notebooks for exploration │ ├── 📄 │ └── ... ├── 📂 scripts/ # Standalone scripts (ETL, data processing) │ ├── 🐍 │ ├── 🐍 │ └── ... ├── 📂 data/ # Raw or processed data (if applicable) │ ├── 📂 raw/ │ ├── 📂 processed/ │ └── ... ├── 📄 . gitignore # Git ignored files ├── 📄 # Project configuration (Poetry, setuptools) ├── 📄 # UV file ├── 📄 # Main project documentation ├── 🐍 # Installation script (if applicable) ├── 📄 LICENSE # Project license ├── 📄 Makefile # Automates common tasks ├── 📄 DockerFile # To create Docker image ├── 📂 . github/ # GitHub Actions workflows (CI/CD) │ ├── 📂 actions/ │ └── 📂 workflows/.
Choosing between these layouts depends on the project’s complexity and. Long-term goals. For production-quality code, the src/ layout is often recommended, whereas the flat layout works well for simple or short-lived projects.
You can imagine different templates that are superior adapted to your use case. It is key that you maintain the modularity of your project. Do not hesitate to create subdirectories and to group together scripts with similar functionalities and separate those with different uses. A good code structure ensures readability, maintainability, scalability and reusability and helps to identify and. Correct errors efficiently.
Cookiecutter is an open-source tool for generating preconfigured project structures from templates. It is particularly useful for ensuring the coherence and organization of projects, especially in Python, by applying good practices from the outset. The flat layout and src layout can be initiate using a UV tool.
SOLID programming is an essential approach to software development based on five basic principles for improving code quality, maintainability and. Scalability. These principles provide a clear framework for developing robust, flexible systems. By following the Solid Principles, you reduce the risk of complex dependencies, make testing easier and. Ensure that applications can evolve more easily in the face of change. Whether you are working on a single project or a large-scale application, mastering SOLID is an essential step towards adopting object-oriented programming best practices.
S — Single Responsibility Principle (SRP).
The principle of single responsibility means that a class/function can only manage one thing. This means that it only has one reason to change. This makes the code more maintainable and easier to read. A class/function with multiple responsibilities is difficult to understand and often a source of errors.
# Violates SRP class MLPipeline: def __init__(self, df: pd. DataFrame, target_column: str): = df self. target_column = target_column = StandardScaler() = RandomForestClassifier() def preprocess_data(self): , inplace=True) # Handle missing values X = [self. target_column]) y = [self. target_column] X_scaled = # Feature scaling return X_scaled, y def train_model(self): X, y = self. preprocess_data() # Data preprocessing inside model training , y) print("Model training complete.").
Here, the research class has two responsibilities: Generate content and save the file.
# Follows SRP class DataPreprocessor: def __init__(self): = StandardScaler() def preprocess(self. Df: pd. DataFrame, target_column: str): df = , inplace=True) # Handle missing values X = [target_column]) # Follows SRP class DataPreprocessor: def __init__(self): = StandardScaler() def preprocess(self. Df: pd. DataFrame, target_column: str): df = , inplace=True) # Handle missing values X = [target_column]) y = df[target_column] X_scaled = # Feature scaling return X_scaled, y class ModelTrainer: def __init__(self, model): = model def train(self, X. Y): , y) print("Model training complete.").
In relation to this, the open/close principle means that a class/function must be open to extension, but closed to modification. This makes it possible to add functionality without the risk of breaking existing code.
It is not easy to develop with this principle in mind, but a good indicator for the main developer is to see more and more additions (+) and fewer and. Fewer removals (-) in the merge requests during project development.
The Liskov substitution principle states that a subordinate class can replace its parent class without changing the behavior of the program, ensuring that the subordinate class meets the expectations defined by the base class. It limits the risk of unexpected errors.
# Violates LSP class Rectangle: def __init__(self, width, height): = width = height def area(self): return * class Square(Rectangle): def __init__(self, side): super().__init__(side, side) # Changing the width of a square violates the idea of a square.
To respect the LSP, it is more effective to avoid this hierarchy and use independent classes:
class Shape: def area(self): raise NotImplementedError class Rectangle(Shape): def __init__(self. Width, height): = width = height def area(self): return * class Square(Shape): def __init__(self, side): = side def area(self): return * .
I — Interface Segregation Principle (ISP).
The principle of interface separation states that several small classes should be built instead of one with methods that cannot be used in certain cases. This reduces unnecessary dependencies.
# Violates ISP class Animal: def fly(self): raise NotImplementedError def swim(self): raise NotImplementedError.
It is advanced to split the class Animal into several classes:
# Follows ISP class CanFly: def fly(self): raise NotImplementedError class CanSwim: def swim(self): raise NotImplementedError class Bird(CanFly): def fly(self): print("Flying") class Fish(CanSwim): def swim(self): print("Swimming").
D — Dependency Inversion Principle (DIP).
The Dependency Inversion Principle means that a class must depend on an abstract class and. Not on a concrete class. This reduces the connections between the classes and makes the code more modular.
# Violates DIP class Database: def connect(self): print("Connecting to database") class UserService: def __init__(self): = Database() def get_users(self): print("Getting people").
Here. The attribute db of UserService depends on the class Database. To respect the DIP, db has to depend on an abstract class.
# Follows DIP class DatabaseInterface: def connect(self): raise NotImplementedError class MySQLDatabase(DatabaseInterface): def connect(self): print("Connecting to MySQL database") class UserService: def __init__(self. Db: DatabaseInterface): = db def get_users(self): print("Getting clients") # We can easily change the used database. db = MySQLDatabase() service = UserService(db) service. get_users().
PEPs (Python Enhancement Proposals) are technical and informative documents that describe new capabilities, language improvements or guidelines for the Python community. Among them, PEP 8, which defines style conventions for Python code, plays a fundamental role in promoting readability and consistency in projects.
Adopting the PEP standards, especially PEP 8. Not only ensures that the code is understandable to other developers, but also that it conforms to the standards set by the community. This facilitates collaboration, re-reads and long-term maintenance.
In this article, I present the most critical aspects of the PEP standards, including:
Style Conventions (PEP 8): Indentations, variable names and import organization.
Best practices for documenting code (PEP 257).
Recommendations for writing typed, maintainable code (PEP 484 and PEP 563).
Understanding and applying these standards is essential to take full advantage of the Python ecosystem and. Contribute to professional quality projects.
This documentation is about coding conventions to standardize the code, and there exists a lot of documentation about the PEP 8. I will not show all recommendation in this posts, only those that I judge essential when I review a code.
Variable, function and module names should be in lower case. And use underscore to separate words. This typographical convention is called snake_case.
Constances are written in capital letters and set at the beginning of the script (after the imports):
Finally, class names and. Exceptions use the CamelCase format (a capital letter at the beginning of each word). Exceptions must contain an Error at the end.
Remember to give your variables names that make sense! Don’t use variable names like v1, v2, func1, i, toto….
Single-character variable names are permitted for loops and indexes:
my_list = [1, 3, 5, 7, 9, 11] for i in range(len(my_liste)): print(my_list[i]).
A more “pythonic” way of writing, to be preferred to the previous example, gets rid of the i index:
my_list = [1, 3, 5, 7, 9, 11] for element in my_list: print(element ).
It is recommended surrounding operators (+, -, *, /. //, %, ==, !=, >, not, in, and, or, …) with a space before AND after:
# recommended code: my_variable = 3 + 7 my_text = "mouse" my_text == my_variable # not recommended code: my_variable=3+7 my_text="mouse" my_text== ma_variable.
You can’t add several spaces around an operator. On the other hand, there are no spaces inside square brackets, braces or parentheses:
# recommended code: my_list[1] my_dict{"key"} my_function(argument) # not recommended code: my_list[ 1 ] my_dict{ "key" } my_function( argument ).
A space is recommended after the characters “:” and “,”, but not before:
# recommended code: my_list = [1, 2, 3] my_dict = {"key1": "value1", "key2": "value2"} my_function(argument1, argument2) # not recommended code: my_list = [1 , 2 , 3] my_dict = {"key1":"value1", "key2":"value2"} my_function(argument1 , argument2).
However. When indexing lists, we don’t put a space after the “:”:
my_list = [1, 3, 5, 7, 9, 1] # recommended code: my_list[1:3] my_list[1:4:2] my_list[::2] # not recommended code: my_list[1 : 3] my_list[1: 4:2 ] my_list[ : :2].
For the sake of readability, we recommend writing lines of code no longer than 80 characters long. However, in certain circumstances this rule can be broken, especially if you are working on a Dash project, it may be complicated to respect this recommendation.
The \ character can be used to cut lines that are too long.
my_variable = 3 if my_variable > 1 and my_variable < 10 \ and my_variable % 2 == 1 and. My_variable % 3 == 0: print(f"My variable is equal to {my_variable }").
Within a parenthesis, you can return to the line without using the \ character. This can be useful for specifying the arguments of a function or method when defining or using it:
def my_function(argument_1, argument_2, argument_3, argument_4): return argument_1 + argument_2.
It is also possible to create multi-line lists or dictionaries by skipping a line after a comma:
my_list = [1, 2, 3, 4, 5, 6. 7, 8, 9] my_dict = {"key1": 13, "key2": 42, "key2": -10}.
Furthermore, in a script, blank lines are useful for visually separating different parts of the code. It is recommended to leave two blank lines before the definition of a function or class, and. To leave a single blank line before the definition of a method (in a class). You can also leave a blank line in the body of a function to separate the logical sections of the function, but. This should be used sparingly.
Comments always begin with the # symbol followed by a space. They give clear explanations of the purpose of the code and must be synchronized with the code. If the code is modified, the comments must be too (if applicable). They are on the same indentation level as the code they comment on. Comments are complete sentences, with a capital letter at the beginning (unless the first word is a variable, which is written without a capital letter) and a period at the strongly recommend writing comments in English and. It is essential to be consistent between the language used for comments and the language used to name variables. Finally, Comments that follow the code on the same line should be avoided wherever possible, and should be separated from the code by at least two spaces.
Ruff is a linter (code analysis tool) and. Formatter for Python code written in Rust. It combines the advantages of the flake8 linter and black and isort formatting while being faster.
Ruff has an extension on the VS Code editor.
But. It is also possible to correct it with the following command:
PEP 20: The Zen of Python is a set of 19 principles written in poetic form. They are more a way of coding than actual guidelines.
Special cases aren’t special enough to break the rules.
In the face of ambiguity, refuse the temptation to guess.
Moving to another aspect, there should be one– and preferably only one –obvious way to do it.
Although that way may not be obvious at first unless you’re Dutch.
Although never is often superior than *right* now.
If the implementation is hard to explain, it’s a bad idea.
If the implementation is easy to explain. It may be a good idea.
Namespaces are one honking great idea — let’s do more of those!
The aim of PEP 257 is to standardize the use of docstrings.
A docstring is a string that appears as the first instruction after the definition of a function, class or method. A docstring becomes the output of the __doc__ special attribute of this object.
def my_function(): """This is a doctring.""" pass.
>>> my_function.__doc__ >>> 'This is a doctring.'
We always write a docstring between triple double quote """ .
Used for simple functions or methods, it must fit on a single line. With no blank line at the beginning or end. The closing quotes are on the same line as opening quotes and there are no blank lines before or after the docstring.
def add(a, b): """Return the sum of a and. B.""" return a + b.
Single-line docstring MUST NOT reintegrate function/method parameters. Do not do:
def my_function(a, b): """ my_function(a, b) -> list"""
Moving to another aspect, the first line should be a summary of the object being documented. An empty line follows, followed by more detailed explanations or clarifications of the arguments.
def divide(a, b): """Divide a byb. Returns the result of the division. Raises a ValueError if b equals 0. """ if b == 0: raise ValueError("Only Chuck Norris can divide by 0") return a / b.
A complete docstring is made up of several parts (in this case. Based on the numpydoc standard).
Short description: Summarizes the main functionality. Parameters: Describes the arguments with their type, name and role. Returns: Specifies the type and role of the returned value. Raises: Documents exceptions raised by the function. Notes (optional): Provides additional explanations. Examples (optional): Contains illustrated usage examples with expected results or exceptions.
def calculate_mean(numbers: list[float]) -> float: """ Calculate the mean of a list of numbers. Parameters ---------- numbers : list of float A list of numerical values for which the mean is to be calculated. Returns ------- float The mean of the input numbers. Raises ------ ValueError If the input list is empty. Notes ----- The mean is calculated as the sum of all elements divided by the number of elements. Examples -------- Calculate the mean of a list of numbers: >>> calculate_mean([, , , ]) """
VsCode’s autoDocstring extension lets you automatically create a docstring template.
In some programming languages. Typing is mandatory when declaring a variable. In Python, typing is optional, but strongly recommended. PEP 484 introduces a typing system for Python, annotating the types of variables, function arguments and return values. This PEP provides a basis for improving code readability, facilitating static analysis and reducing errors.
Typing consists in explicitly declaring the type (float. String, etc.) of a variable. The typing module provides standard tools for defining generic types, such as Sequence, List, Union, Any, etc.
To type function attributes, we use “:” for function arguments and “->” for the type of what is returned.
def show_message(message): print(f"Message : {message}") def addition(a, b): return a + b def is_even(n): return n % 2 == 0 def list_square(numbers): return [x**2 for x in numbers] def reverse_dictionary(d): return {v: k for k, v in } def add_element(ensemble, element): return ensemble.
from typing import List, Tuple, Dict, Set. Any def show_message(message: str) -> None: print(f"Message : {message}") def addition(a: int, b: int) -> int: return a + b def is_even(n: int) -> bool: return n % 2 == 0 def list_square(numbers: List[int]) -> List[int]: return [x**2 for x in numbers] def reverse_dictionary(d: Dict[str, int]) -> Dict[int, str]: return {v: k for k, v in } def add_element(ensemble: Set[int], element: int) -> Set[int]: return ensemble.
Moving to another aspect, the MyPy extension automatically checks whether the use of a variable corresponds to the declared type. For example, for the following function:
def my_function(x: float) -> float: return .
In relation to this, the editor will point out that a float has no “mean” attribute.
The benefit is twofold: you’ll know whether the declared type is the right one and whether the use of this variable corresponds to its type.
In the above example. X must be of a type that has a mean() method ( .
In this article, we have looked at the most essential principles for creating clean Python production code. A solid architecture, adherence to SOLID principles, and compliance with PEP recommendations (at least the four discussed here) are essential for ensuring code quality. The desire for beautiful code is not (just) coquetry. It standardizes development practices and makes teamwork and maintenance much easier. There’s nothing more frustrating than spending hours (or even days) reverse-engineering a program, deciphering poorly written code before you’re finally able to fix the bugs. By applying these best practices, you ensure that your code remains clear, scalable, and easy for any developer to work with in the future.
During a press conference at the White House Monday, US President Donald Trump and. Taiwan Semiconductor Manufactur...
How well are investments understood today? If asked, many parents would likely suggest traditional investment options like a fixed deposit or gold. Wh...
SpaceTech startup TakeMe2Space has secured ₹ crore in a pre-seed funding round led by Seafund, with participation from Artha Venture Fund, Blume Ve...
Jio Platforms to Build Open Telecom AI Platform with AMD, Cisco and Nokia
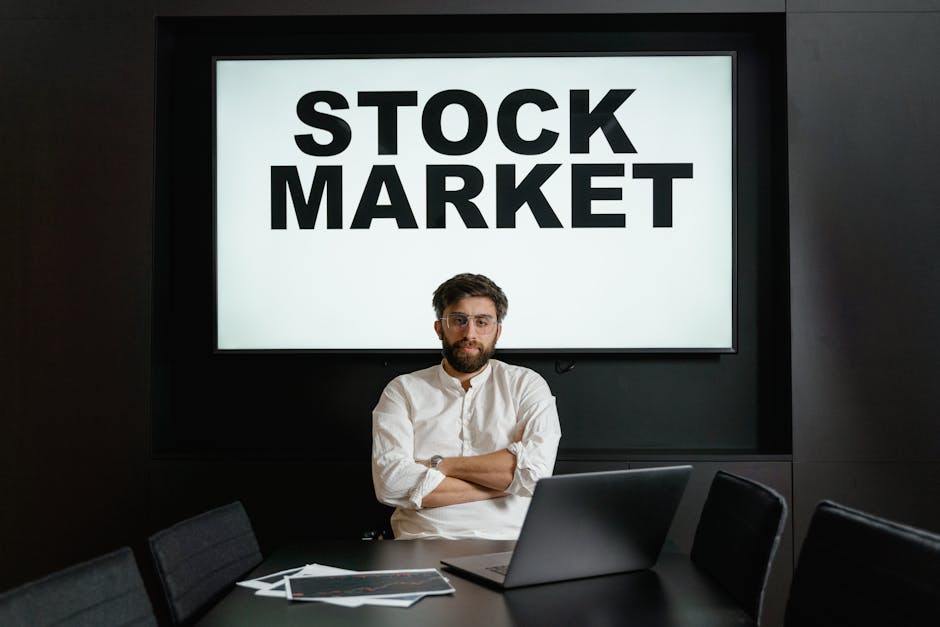
At the Mobile World Congress (MWC) 2025 held in Barcelona, Jio Platforms Limited (JPL), along with AMD, Cisco, and Nokia. On Monday unveiled that it plans to develop an Open Telecom AI Platform.
The platform offers a ‘multi-domain intelligence’ framework to introduce AI and automation to ‘every layer’ of network operations. It will utilise open APIs, agentic AI and harness various large, and domain-specific small language models to enable end-to-end intelligence for network management and operations.
“This initiative goes beyond automation—it’s about enabling AI-driven, autonomous networks that adapt in real time, enhance user experiences, and create new service and revenue opportunities across the digital ecosystem,” introduced Mathew Oommen, Group CEO of JPL.
The platform will use AMD’s portfolio of high performance CPUs, GPUs, and other computing solutions.
“AMD is proud to collaborate with Jio Platforms Limited, Cisco. And Nokia to power the next generation of AI-driven telecom infrastructure,” introduced Lisa Su, CEO, of AMD.
Cisco is set to integrate its data center, analytics, security, and networking solutions into the platform. On the other hand, Nokia will bring its experience in domains such as broadband, optical transport, RAN and more to the platform.
“The Telecom AI Platform will help Jio to optimise and monetise their network investments through enhanced performance, security, operational efficiency, automation and greatly improved customer experience, all via the immense power of artificial intelligence,” expressed Pekka Lundmark, CEO of Nokia.
The Open Telecom AI platform will be built with Jio as the first customer, which is set to create ‘a replicable reference architecture and deployable solution for the broader global service provider industry.’.
in recent times, California-based Confluent, the data streaming giant founded by the creators of Apache Kafka. revealed a strategic partnership with Jio Platforms Limited to integrate its data streaming platform with Jio Cloud Services.
Furthermore, this agreement positions Confluent as the first data streaming platform available on Jio Cloud.
Argenteuil s’impose comme un pôle central pour la formation en intelligence artificielle, combinant innovation technologique et opportunités de dévelo...
India’s AI landscape is witnessing rapid growth, however, access to early-stage capital remains challenging. While large venture capital (VC) firms an...
Hyderabad is rapidly establishing itself as a major life sciences hub, rivaling Bengaluru. Thanks to a robust state-supported business ecosystem attra...
Google Releases Data Science Agent in Colab
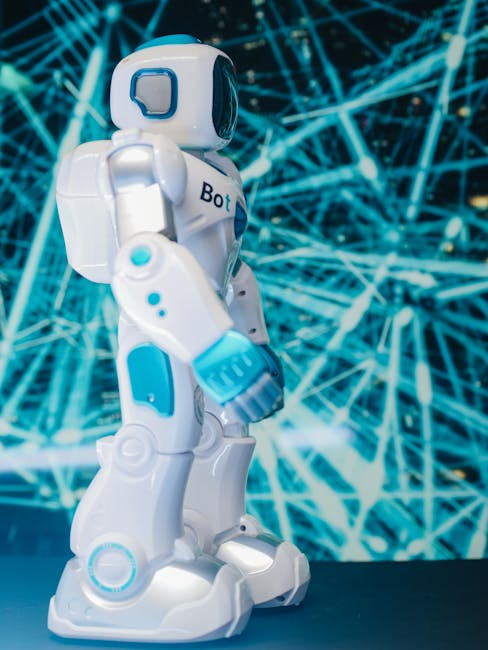
Google released a Data Science Agent on the Colab platform on Monday, powered by its Gemini AI model. The Data Science Agent is capable of autonomously generating the required analysis of the data file uploaded by the user. It is also capable of creating fully functional notebooks, and not just code snippets.
Google mentioned the agent “removes tedious setup tasks like importing libraries, loading data. And writing boilerplate code”. The agent achieves goals set by the user by “orchestrating a composite flow” which mimics the workflow of a typical data scientist. individuals can use the agent to clean data, perform exploratory data analysis, statistical analysis, predictive modeling and. Other such tasks.
The generated code can be customised and extended to meet individuals’ needs. Moreover, results can also be shared with other developers on Colab. Google also stated that the agent ranked fourth on the DAPStep (Data Agent Benchmark) on HuggingFace, ahead of GPT-4o, DeepSeek-V3, Llama 70B and. More.
The Data Science Agent was launched for trusted testers last December, but is now available on Google Colab. Colab is a free, cloud-based environment where Python code can be written and run within the web browser. It also provides free access to Google Cloud GPUs and TPUs.
“We want to simplify and automate common data science tasks like predictive modelling, data preprocessing, and. Visualisation,” Google noted.
in recent times, Google also revealed the public preview of Gemini Code Assist, a free AI-powered coding assistant for individuals. The tool is globally available and supports all programming languages in the public domain.
It is available in Visual Studio (VS) Code and JetBrains IDEs. As well as in Firebase and Android Studio. Google also expressed the AI coding assistant offers “practically unlimited capacity with up to 1,80,000 code completions per month”.
Klarna CEO Sebastian Siemiatkowski noted he was “tremendously embarrassed” after the business’s decision to move away from Salesforce and other SaaS pro...
Shenzhen-based UBTECH Robotics has successfully completed what is claimed to be the world’s first multi-humanoid robot collaborative training program ...
L’IA devient un outil incontournable. Mais elle laisse encore des indices derrière elle. ChatGPT, développé par OpenAI, produit des textes généralemen...
Market Impact Analysis
Market Growth Trend
2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|
23.1% | 27.8% | 29.2% | 32.4% | 34.2% | 35.2% | 35.6% |
Quarterly Growth Rate
Q1 2024 | Q2 2024 | Q3 2024 | Q4 2024 |
---|---|---|---|
32.5% | 34.8% | 36.2% | 35.6% |
Market Segments and Growth Drivers
Segment | Market Share | Growth Rate |
---|---|---|
Machine Learning | 29% | 38.4% |
Computer Vision | 18% | 35.7% |
Natural Language Processing | 24% | 41.5% |
Robotics | 15% | 22.3% |
Other AI Technologies | 14% | 31.8% |
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity:
Competitive Landscape Analysis
Company | Market Share |
---|---|
Google AI | 18.3% |
Microsoft AI | 15.7% |
IBM Watson | 11.2% |
Amazon AI | 9.8% |
OpenAI | 8.4% |
Future Outlook and Predictions
The Data Science From landscape is evolving rapidly, driven by technological advancements, changing threat vectors, and shifting business requirements. Based on current trends and expert analyses, we can anticipate several significant developments across different time horizons:
Year-by-Year Technology Evolution
Based on current trajectory and expert analyses, we can project the following development timeline:
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity, influencing adoption timelines and investment priorities:
Innovation Trigger
- Generative AI for specialized domains
- Blockchain for supply chain verification
Peak of Inflated Expectations
- Digital twins for business processes
- Quantum-resistant cryptography
Trough of Disillusionment
- Consumer AR/VR applications
- General-purpose blockchain
Slope of Enlightenment
- AI-driven analytics
- Edge computing
Plateau of Productivity
- Cloud infrastructure
- Mobile applications
Technology Evolution Timeline
- Improved generative models
- specialized AI applications
- AI-human collaboration systems
- multimodal AI platforms
- General AI capabilities
- AI-driven scientific breakthroughs
Expert Perspectives
Leading experts in the ai tech sector provide diverse perspectives on how the landscape will evolve over the coming years:
"The next frontier is AI systems that can reason across modalities and domains with minimal human guidance."
— AI Researcher
"Organizations that develop effective AI governance frameworks will gain competitive advantage."
— Industry Analyst
"The AI talent gap remains a critical barrier to implementation for most enterprises."
— Chief AI Officer
Areas of Expert Consensus
- Acceleration of Innovation: The pace of technological evolution will continue to increase
- Practical Integration: Focus will shift from proof-of-concept to operational deployment
- Human-Technology Partnership: Most effective implementations will optimize human-machine collaboration
- Regulatory Influence: Regulatory frameworks will increasingly shape technology development
Short-Term Outlook (1-2 Years)
In the immediate future, organizations will focus on implementing and optimizing currently available technologies to address pressing ai tech challenges:
- Improved generative models
- specialized AI applications
- enhanced AI ethics frameworks
These developments will be characterized by incremental improvements to existing frameworks rather than revolutionary changes, with emphasis on practical deployment and measurable outcomes.
Mid-Term Outlook (3-5 Years)
As technologies mature and organizations adapt, more substantial transformations will emerge in how security is approached and implemented:
- AI-human collaboration systems
- multimodal AI platforms
- democratized AI development
This period will see significant changes in security architecture and operational models, with increasing automation and integration between previously siloed security functions. Organizations will shift from reactive to proactive security postures.
Long-Term Outlook (5+ Years)
Looking further ahead, more fundamental shifts will reshape how cybersecurity is conceptualized and implemented across digital ecosystems:
- General AI capabilities
- AI-driven scientific breakthroughs
- new computing paradigms
These long-term developments will likely require significant technical breakthroughs, new regulatory frameworks, and evolution in how organizations approach security as a fundamental business function rather than a technical discipline.
Key Risk Factors and Uncertainties
Several critical factors could significantly impact the trajectory of ai tech evolution:
Organizations should monitor these factors closely and develop contingency strategies to mitigate potential negative impacts on technology implementation timelines.
Alternative Future Scenarios
The evolution of technology can follow different paths depending on various factors including regulatory developments, investment trends, technological breakthroughs, and market adoption. We analyze three potential scenarios:
Optimistic Scenario
Responsible AI driving innovation while minimizing societal disruption
Key Drivers: Supportive regulatory environment, significant research breakthroughs, strong market incentives, and rapid user adoption.
Probability: 25-30%
Base Case Scenario
Incremental adoption with mixed societal impacts and ongoing ethical challenges
Key Drivers: Balanced regulatory approach, steady technological progress, and selective implementation based on clear ROI.
Probability: 50-60%
Conservative Scenario
Technical and ethical barriers creating significant implementation challenges
Key Drivers: Restrictive regulations, technical limitations, implementation challenges, and risk-averse organizational cultures.
Probability: 15-20%
Scenario Comparison Matrix
Factor | Optimistic | Base Case | Conservative |
---|---|---|---|
Implementation Timeline | Accelerated | Steady | Delayed |
Market Adoption | Widespread | Selective | Limited |
Technology Evolution | Rapid | Progressive | Incremental |
Regulatory Environment | Supportive | Balanced | Restrictive |
Business Impact | Transformative | Significant | Modest |
Transformational Impact
Redefinition of knowledge work, automation of creative processes. This evolution will necessitate significant changes in organizational structures, talent development, and strategic planning processes.
The convergence of multiple technological trends—including artificial intelligence, quantum computing, and ubiquitous connectivity—will create both unprecedented security challenges and innovative defensive capabilities.
Implementation Challenges
Ethical concerns, computing resource limitations, talent shortages. Organizations will need to develop comprehensive change management strategies to successfully navigate these transitions.
Regulatory uncertainty, particularly around emerging technologies like AI in security applications, will require flexible security architectures that can adapt to evolving compliance requirements.
Key Innovations to Watch
Multimodal learning, resource-efficient AI, transparent decision systems. Organizations should monitor these developments closely to maintain competitive advantages and effective security postures.
Strategic investments in research partnerships, technology pilots, and talent development will position forward-thinking organizations to leverage these innovations early in their development cycle.
Technical Glossary
Key technical terms and definitions to help understand the technologies discussed in this article.
Understanding the following technical concepts is essential for grasping the full implications of the security threats and defensive measures discussed in this article. These definitions provide context for both technical and non-technical readers.