Goodbye Create React App, Hello TanStack Create React App - Related to build, document, summarization, deprecated!, hello
Build a Local AI-Powered Document Summarization Tool
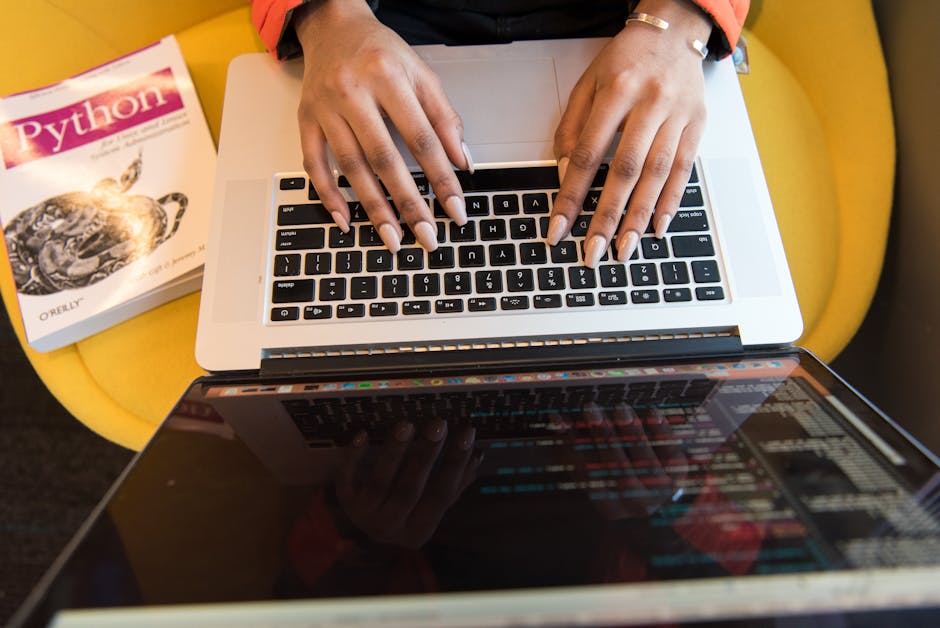
When I began my journey into the field of AI and large language models (LLMs), my initial aim was to experiment with various models and learn about their effectiveness. Like most developers, I also began using cloud-hosted services, enticed by the ease of quick setup and availability of ready-to-use LLMs at my fingertips.
But pretty quickly, I ran into a snag: cost. It is convenient to use LLMs in the cloud, but the pay-per-token model can suddenly get really expensive, especially when working with lots of text or asking many questions. It made me realize I needed a improved way to learn and experiment with AI without blowing my budget. This is where Ollama came in, and it offered a rather interesting solution.
Load and experiment with multiple LLMs locally.
Avoid API rate limits and usage restrictions.
In this article, we will explore how to build a simple document summarization tool using Ollama, Streamlit, and LangChain. Ollama allows us to run LLMs locally, Streamlit provides a web interface so that clients may interact with those models smoothly, and LangChain offers pre-built chains for simplified development.
Ensure Python [website] or higher is installed.
Fetch [website] model via ollama run [website].
I prefer to use Conda for managing dependencies and creating isolated environments. Create a new Conda environment and then install the necessary packages mentioned below.
Shell pip install streamlit langchain langchain-ollama langchain-community langchain-core pymupdf.
Now, let's dive into building our document summarizer. We will start by creating a Streamlit app to handle uploading documents and displaying summaries in a user-friendly interface.
Next, we will focus on pulling the text out of the uploaded documents (supports only PDF and text documents) and preparing everything for the summarization chain.
Finally, we will bring Ollama to actually perform the summarization utilizing its local language model capabilities to generate concise and informative summaries.
The code below contains the complete implementation, with detailed comments to guide you through each step.
Python import os import tempfile import streamlit as stlit from langchain_text_splitters import CharacterTextSplitter from [website] import load_summarize_chain from langchain_ollama import OllamaLLM from langchain_community.document_loaders import PyMuPDFLoader from langchain_core.documents import Document # Create Streamlit app by page configuration, title and a file uploader stlit.set_page_config(page_title="Local Document Summarizer", layout="wide") [website]"Local Document Summarizer") # File uploader that accepts pdf and txt files only uploaded_file = stlit.file_uploader("Choose a PDF or Text file", type=["pdf", "txt"]) # Process the uploaded file and extracts text from it def process_file(uploaded_file): if [website]".pdf"): with tempfile.NamedTemporaryFile(delete=False) as temp_file: [website] loader = PyMuPDFLoader([website] docs = [website] extracted_text = " ".join([doc.page_content for doc in docs]) [website] else: # Read the content directly for text files, no need for tempfile extracted_text = uploaded_file.getvalue().decode("utf-8") return extracted_text # Process the extracted text and return summary def summarize(text): # Split the text into chunks for processing and create Document object chunks = CharacterTextSplitter(chunk_size=500, chunk_overlap=100).split_text(text) docs = [Document(page_content=chunk) for chunk in chunks] # Initialize the LLM with [website] model and load the summarization chain chain = load_summarize_chain(OllamaLLM(model="[website]"), chain_type="map_reduce") return [website] if uploaded_file: # Process and preview the uploaded file content extracted_text = process_file(uploaded_file) stlit.text_area("Document Preview", extracted_text[:1200], height=200) # Generate a summary of the extracted text if [website]"Generate Summary"): with stlit.spinner("[website] take a few seconds"): summary_text = summarize(extracted_text) stlit.text_area("Summary", summary_text['output_text'], height=400).
Save the above code snippet into [website] , then open your terminal, navigate to where you saved the file, and run:
That should start your Streamlit app and automatically open in your web browser, pointing to a local URL like [website]:8501.
You've just completed the document summarization tool by combining Streamlit’s simplicity and Ollama’s local model hosting capabilities. This example utilizes the [website] model, but you can experiment with other models to determine what is best for your needs, and you can also consider adding support for additional document formats, error handling, and customized summarization parameters.
Time To First Byte: Beyond Server Response Time.
Optimizing web performance means looking beyond surface-level......
The goal of content design is to reduce confusion and improve clarity. ......
You know about Baseline, right? And you may have heard that the Chrome team made a web component for it.
Goodbye Create React App, Hello TanStack Create React App
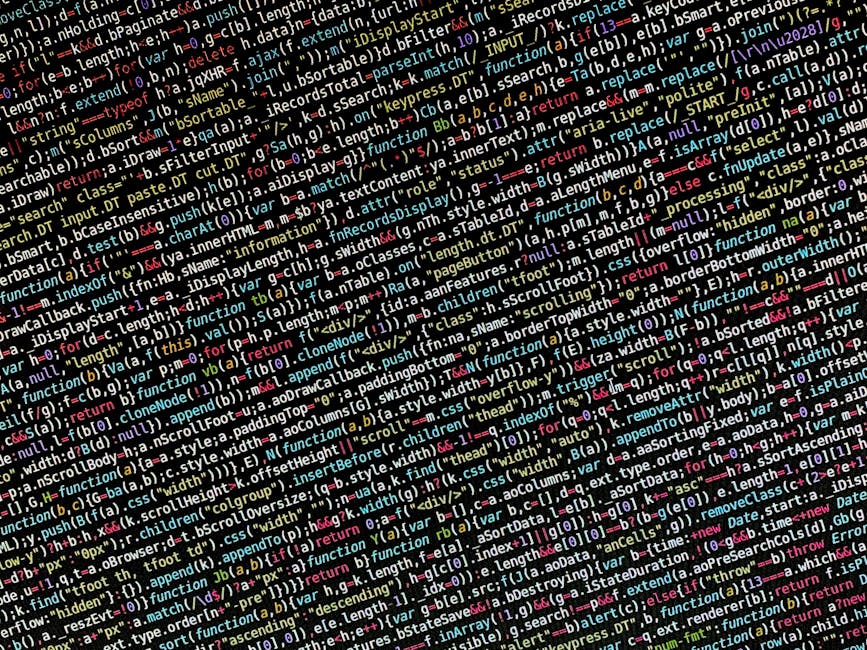
Create React App was deprecated Feb. 14 after nearly a decade of use building React apps. It will continue working in maintenance mode.
“Today, we’re deprecating Create React App for new apps, and encouraging existing apps to migrate to a framework, or to migrate to a build tool like Vite, Parcel, or Rsbuild,” wrote Meta’s Matt Carroll and Rickey Hanlon.
The Create React App (CRA) library was released in 2016 when there was no clear way to build a new React app, the two wrote. It combined several tools into a single recommended configuration to simplify app development, allowing developers to quickly spin up a React project. It included a basic file structure for the website and a development server to run the website locally for easy development.
“This allowed apps a simple way to upgrade to new tooling elements, and allowed the React team to deploy non-trivial tooling changes (Fast Refresh support, React Hooks lint rules) to the broadest possible audience,” they wrote. “This model became so popular that there’s an entire category of tools working this way today.”.
The blog post outlined CRA’s problems, including that it’s difficult to build high performant production apps. It also noted that Create React App does not offer specific options for routing, data fetching or code splitting.
“In principle, we could solve these problems by essentially evolving it into a framework,” they wrote.
But that raises what may be the biggest challenge for CRA: It has null active maintainers.
So, the team is recommending that developers create new React apps with a framework.
“All the frameworks we recommend support client-side rendering (CSR) and single-page apps (SPA), and can be deployed to a CDN or static hosting service without a server,” they added.
They offer links to migration guides for [website], React Router and the Expo web pack to Expo Router.
“If your app has unusual constraints, or you prefer to solve these problems by building your own framework, or you just want to learn how React works from scratch, you can roll your own custom setup with React using Vite, Parcel or Rsbuild,” they added.
The in recent times released 2024 State of React ranked CRA as the third most-used tool, behind the Fetch API and useState. Eighty-nine percent of the 6,240 developers who responded about CRA had used the tool, but from that group nearly 30% reported a negative sentiment about it. Only 15% expressed a positive sentiment. Forty-four percent of frontend developers expressed no sentiment.
New Create React App for TanStack Router.
In related news, TanStack not long ago added an open source Create React App for TanStack Router. It’s designed to be a drop-in replacement for Create React App. This will allow developers to build SPA applications based on their Router.
To help accelerate the migration away from create-react-app, the team has created the create-tsrouter-app CLI, which is a plug-n-play replacement for CRA.
“What you’ll get is a Vite application that uses TanStack Router,” the project notes state. “create-tsrouter-app is everything you loved about CR but implemented with modern tools and best practices, on top of the popular TanStack set of libraries.”.
That includes Tanstack Query, an asynchronous state management for TS/JS, React, Solid, Vue, Svelte and Angular, and Tanstack Router for React and Solid applications.
Anaconda introduced a new open source AI data tool on Wednesday that lets data science and development teams explore, transform and visualize data using natural language.
It’s called Lumen AI and it’s an agent-based framework for “chatting with data” and retrieval augmented generation (RAG). The goal is to make advanced data workflows more intuitive and scalable, .
“AI-driven, agent-based systems are rapidly changing how businesses operate, but many organizations still struggle with technical barriers, fragmented tools, and slow, manual processes,” Kodie Dower, senior marketing communications manager, wrote. “Lumen eliminates those roadblocks by giving people an AI-powered environment to quickly generate SQL queries, analyze datasets, and build interactive dashboards — all without writing code.”.
Create visualizations such as charts, tables and dashboards without coding;
Generate SQL queries and transform data across local files, databases, and cloud data lakes;
Support collaborations with serialized and shared workflows;
Inspect, validate and edit AI-generated outputs to ensure data accuracy and clarity;
“The declarative nature of Lumen’s data model makes it possible for LLMs to easily generate entire data transformation pipelines, visualizations and many other types of output,” the repository explained. “Once generated the data pipelines and visual output can be easily serialized, making it possible to share them, to continue the analysis in a notebook and/or build entire dashboards.”.
Vercel Adds Support for React Router v7 Apps.
React Router version 7 is a bit different than its previous iterations in that it’s also a framework after being merged with Remix. This week, Vercel showcased it will support React Router v7 applications when used as a framework. This includes support for server-rendered React Router applications using Vercel’s Fluid compute.
This article has been updated from when it was originally , 2023.
In today’s highly digital world, we generate tons of data daily......
Look Closer, Inspiration Lies Everywhere (February 2025 Wallpapers Edition).
In modern application development, the need for real-time data processing and reactive programming has become increasingly significant. Reactive program......
Create React App is Being Deprecated!
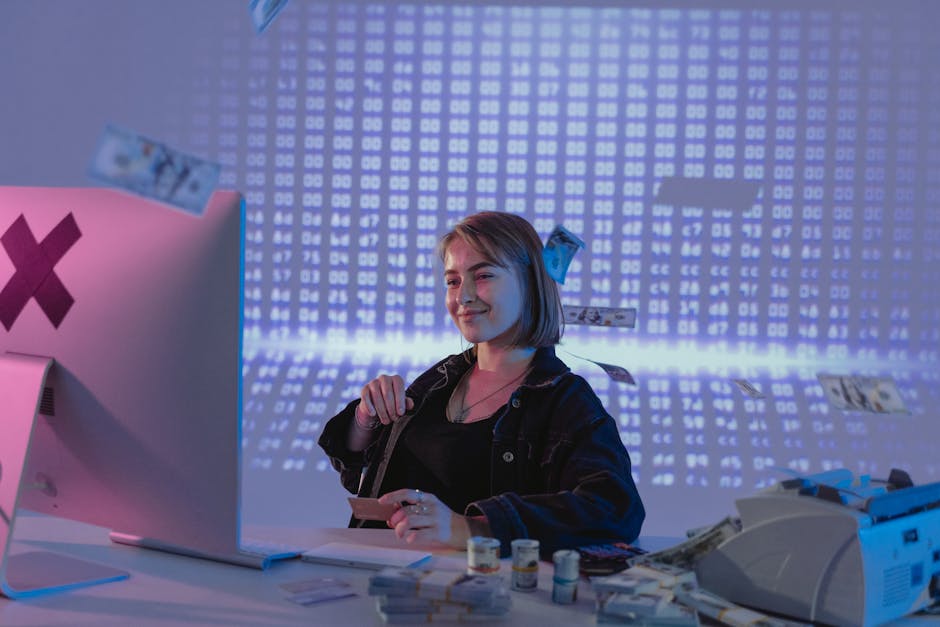
In a recent announcement on the React blog, the React team shared a significant upgrade: Create React App (CRA) is being deprecated. This decision marks the end of an era for one of the most popular tools for bootstrapping React applications. If you’re a React developer, this news is critical—it signals a shift in how the React ecosystem is evolving and what tools you should use moving forward.
In this article, we’ll break down the key points from the blog post, explore why this decision was made, and discuss what it means for your current and future projects.
The React team has officially unveiled that Create React App (CRA) is being deprecated for new projects. While CRA will continue to work in maintenance mode, it will no longer be actively developed or recommended for new apps. Instead, the React team encourages developers to migrate to modern frameworks like [website], React Router, or Expo, or to use build tools like Vite, Parcel, or Rsbuild.
Deprecation Warning: Starting now, if you try to create a new app with CRA, you’ll see a deprecation warning.
Starting now, if you try to create a new app with CRA, you’ll see a deprecation warning. Maintenance Mode: CRA will still work and has been updated to support React 19 , but it won’t receive new functions or improvements.
CRA will still work and has been updated to support , but it won’t receive new capabilities or improvements. Migration Guides: The React team has provided detailed guides for migrating to frameworks or build tools.
CRA was introduced in 2016 to solve a major pain point for React developers: setting up a new React app was complicated and error-prone. Developers had to manually configure tools for JSX, linting, and hot reloading, which led to fragmented boilerplates and inconsistent setups. CRA provided a standardized, zero-configuration solution that made it easy to get started with React.
However, over time, CRA’s limitations became apparent. While it was great for beginners and small projects, it struggled to meet the demands of modern, production-grade applications. Here are some of the key reasons for its deprecation:
CRA doesn’t include solutions for routing, data fetching, or code splitting, which are essential for building performant apps. Developers had to rely on external libraries and custom configurations, which often led to inefficiencies and complexity.
CRA no longer has active maintainers, making it difficult to address issues or add new functions. This lack of support has made it less viable for modern development.
Modern frameworks like [website], React Router, and Expo provide integrated solutions for routing, data fetching, and performance optimization. These frameworks offer a enhanced developer experience and are enhanced suited for production apps.
If you’re currently using CRA or planning to start a new React project, here’s what you need to know:
Use a Framework: The React team recommends using frameworks like [website] , React Router , or Expo . These tools provide built-in solutions for routing, data fetching, and performance optimization.
The React team recommends using frameworks like , , or . These tools provide built-in solutions for routing, data fetching, and performance optimization. Explore Build Tools: If you prefer more control over your setup, consider using build tools like Vite, Parcel, or Rsbuild.
Migrate to a Framework: If your app is growing in complexity, consider migrating to a framework. The React team has provided migration guides for [website] , React Router , and Expo .
If your app is growing in complexity, consider migrating to a framework. The React team has provided migration guides for , , and . Switch to a Build Tool: If a framework isn’t a good fit, you can migrate to a build tool like Vite or Parcel. These tools offer more flexibility and superior performance than CRA.
If you’re interested in understanding how React works under the hood, the React team has . This is a great way to deepen your knowledge and gain more control over your projects.
Frameworks like [website] and React Router are designed to solve the challenges that CRA couldn’t address. They provide integrated solutions for:
Routing: Built-in routing solutions make it easy to structure your app and share links.
Built-in routing solutions make it easy to structure your app and share links. Data Fetching: Frameworks optimize data fetching to avoid network waterfalls and improve performance.
Frameworks optimize data fetching to avoid network waterfalls and improve performance. Code Splitting: Advanced code splitting ensures consumers only download the code they need, reducing load times.
Advanced code splitting ensures clients only download the code they need, reducing load times. Server-Side Rendering (SSR): Frameworks make it easy to implement SSR, which improves performance and SEO.
By using a framework, you can focus on building your app instead of solving tooling and configuration issues.
The deprecation of Create React App is a significant moment for the React ecosystem. While CRA served its purpose well for many years, the demands of modern web development have outgrown its capabilities. By migrating to frameworks or build tools, you can take advantage of more effective performance, more attributes, and a smoother developer experience.
If you’re still using CRA, now is the time to start planning your migration. Check out the official React blog post.
Adam’s such a mad scientist with CSS. He’s been putting together a series of “notebooks” that make it easy for him to demo code. He’s got one for grad......
The Digital Playbook: A Crucial Counterpart To Your Design System.
Design systems play a crucial role in today......
Large Language Model (LLM)-based Generative AI services such as OpenAI or Google Gemini are superior at some tasks more so than others, advised Wei-Meng......
Market Impact Analysis
Market Growth Trend
2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|
7.5% | 9.0% | 9.4% | 10.5% | 11.0% | 11.4% | 11.5% |
Quarterly Growth Rate
Q1 2024 | Q2 2024 | Q3 2024 | Q4 2024 |
---|---|---|---|
10.8% | 11.1% | 11.3% | 11.5% |
Market Segments and Growth Drivers
Segment | Market Share | Growth Rate |
---|---|---|
Enterprise Software | 38% | 10.8% |
Cloud Services | 31% | 17.5% |
Developer Tools | 14% | 9.3% |
Security Software | 12% | 13.2% |
Other Software | 5% | 7.5% |
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity:
Competitive Landscape Analysis
Company | Market Share |
---|---|
Microsoft | 22.6% |
Oracle | 14.8% |
SAP | 12.5% |
Salesforce | 9.7% |
Adobe | 8.3% |
Future Outlook and Predictions
The Create React Build landscape is evolving rapidly, driven by technological advancements, changing threat vectors, and shifting business requirements. Based on current trends and expert analyses, we can anticipate several significant developments across different time horizons:
Year-by-Year Technology Evolution
Based on current trajectory and expert analyses, we can project the following development timeline:
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity, influencing adoption timelines and investment priorities:
Innovation Trigger
- Generative AI for specialized domains
- Blockchain for supply chain verification
Peak of Inflated Expectations
- Digital twins for business processes
- Quantum-resistant cryptography
Trough of Disillusionment
- Consumer AR/VR applications
- General-purpose blockchain
Slope of Enlightenment
- AI-driven analytics
- Edge computing
Plateau of Productivity
- Cloud infrastructure
- Mobile applications
Technology Evolution Timeline
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
Expert Perspectives
Leading experts in the software dev sector provide diverse perspectives on how the landscape will evolve over the coming years:
"Technology transformation will continue to accelerate, creating both challenges and opportunities."
— Industry Expert
"Organizations must balance innovation with practical implementation to achieve meaningful results."
— Technology Analyst
"The most successful adopters will focus on business outcomes rather than technology for its own sake."
— Research Director
Areas of Expert Consensus
- Acceleration of Innovation: The pace of technological evolution will continue to increase
- Practical Integration: Focus will shift from proof-of-concept to operational deployment
- Human-Technology Partnership: Most effective implementations will optimize human-machine collaboration
- Regulatory Influence: Regulatory frameworks will increasingly shape technology development
Short-Term Outlook (1-2 Years)
In the immediate future, organizations will focus on implementing and optimizing currently available technologies to address pressing software dev challenges:
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
These developments will be characterized by incremental improvements to existing frameworks rather than revolutionary changes, with emphasis on practical deployment and measurable outcomes.
Mid-Term Outlook (3-5 Years)
As technologies mature and organizations adapt, more substantial transformations will emerge in how security is approached and implemented:
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
This period will see significant changes in security architecture and operational models, with increasing automation and integration between previously siloed security functions. Organizations will shift from reactive to proactive security postures.
Long-Term Outlook (5+ Years)
Looking further ahead, more fundamental shifts will reshape how cybersecurity is conceptualized and implemented across digital ecosystems:
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
These long-term developments will likely require significant technical breakthroughs, new regulatory frameworks, and evolution in how organizations approach security as a fundamental business function rather than a technical discipline.
Key Risk Factors and Uncertainties
Several critical factors could significantly impact the trajectory of software dev evolution:
Organizations should monitor these factors closely and develop contingency strategies to mitigate potential negative impacts on technology implementation timelines.
Alternative Future Scenarios
The evolution of technology can follow different paths depending on various factors including regulatory developments, investment trends, technological breakthroughs, and market adoption. We analyze three potential scenarios:
Optimistic Scenario
Rapid adoption of advanced technologies with significant business impact
Key Drivers: Supportive regulatory environment, significant research breakthroughs, strong market incentives, and rapid user adoption.
Probability: 25-30%
Base Case Scenario
Measured implementation with incremental improvements
Key Drivers: Balanced regulatory approach, steady technological progress, and selective implementation based on clear ROI.
Probability: 50-60%
Conservative Scenario
Technical and organizational barriers limiting effective adoption
Key Drivers: Restrictive regulations, technical limitations, implementation challenges, and risk-averse organizational cultures.
Probability: 15-20%
Scenario Comparison Matrix
Factor | Optimistic | Base Case | Conservative |
---|---|---|---|
Implementation Timeline | Accelerated | Steady | Delayed |
Market Adoption | Widespread | Selective | Limited |
Technology Evolution | Rapid | Progressive | Incremental |
Regulatory Environment | Supportive | Balanced | Restrictive |
Business Impact | Transformative | Significant | Modest |
Transformational Impact
Technology becoming increasingly embedded in all aspects of business operations. This evolution will necessitate significant changes in organizational structures, talent development, and strategic planning processes.
The convergence of multiple technological trends—including artificial intelligence, quantum computing, and ubiquitous connectivity—will create both unprecedented security challenges and innovative defensive capabilities.
Implementation Challenges
Technical complexity and organizational readiness remain key challenges. Organizations will need to develop comprehensive change management strategies to successfully navigate these transitions.
Regulatory uncertainty, particularly around emerging technologies like AI in security applications, will require flexible security architectures that can adapt to evolving compliance requirements.
Key Innovations to Watch
Artificial intelligence, distributed systems, and automation technologies leading innovation. Organizations should monitor these developments closely to maintain competitive advantages and effective security postures.
Strategic investments in research partnerships, technology pilots, and talent development will position forward-thinking organizations to leverage these innovations early in their development cycle.
Technical Glossary
Key technical terms and definitions to help understand the technologies discussed in this article.
Understanding the following technical concepts is essential for grasping the full implications of the security threats and defensive measures discussed in this article. These definitions provide context for both technical and non-technical readers.