Project Loom vs. Traditional Threads: Java Concurrency Revolution - Related to managing, traditional, concurrency, vs., java
How to Quarantine a Malicious File in Java
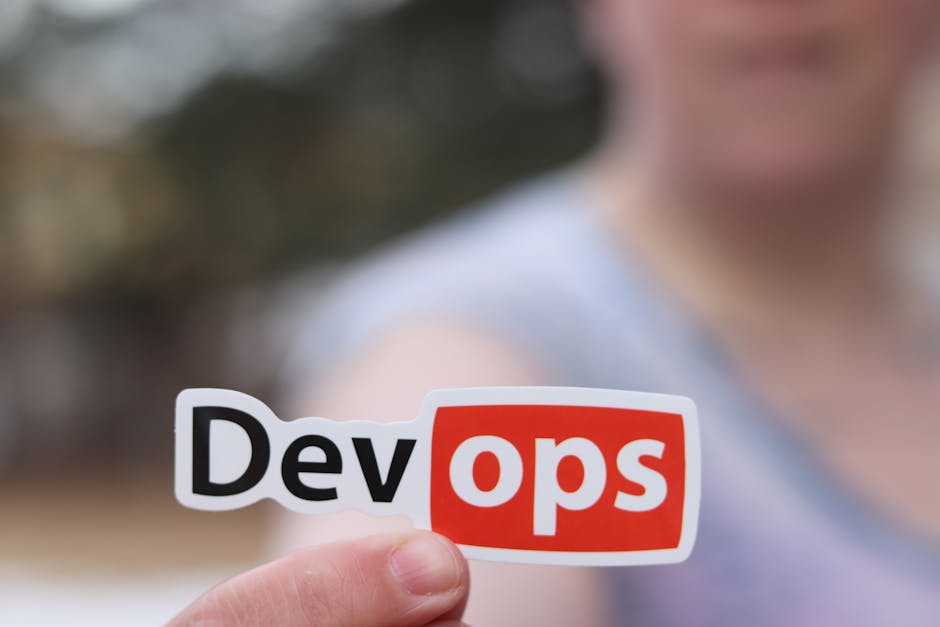
Scanning file uploads for viruses, malware, and other threats is standard practice in any application that processes files from an external source.
No matter which antimalware we use, the goal is always the same: to prevent malicious executables from reaching a downstream user (directly, via database storage, etc.) or automated workflow that might inadvertently execute the malicious content.
In this article, we’ll discuss the value of quarantining malicious files after they’re flagged by an antimalware solution instead of outright deleting them. We’ll highlight several APIs Java developers can leverage to quarantine malicious content seamlessly in their application workflow.
Deleting vs. Quarantining Malicious Files.
While there’s zero debate around whether external files should be scanned for malicious content, there’s a bit more room for debate around how malicious files should be handled once antimalware policies flag them.
The simplest (and overall safest) option is to programmatically delete malicious files as soon as they’re flagged. The logic for deleting a threat is straightforward: it completely removes the possibility that downstream individuals or processes might unwittingly execute the malicious content. If our antimalware false positive rate is extremely low — which it ideally should be — we don’t need to spend too much time debating whether the file in question was misdiagnosed. We can shoot first and ask questions later.
When we elect to programmatically quarantine malicious files, we take on risk in an already-risky situation — but that risk can yield significant rewards. If we can safely contain a malicious file within an isolated directory ([website], a secure zip archive), we can preserve the opportunity to analyze the threat and gain valuable insights from it. This is a bit like sealing a potentially venomous snake in a glass container; with a closer look, we can find out if the snake is truly dangerous, misidentified, or an entirely unique specimen that demands further study to adequately understand.
In quarantining a malicious file, we might be preserving the latest enhancement of some well-known and oft-employed black market malware library, or in cases involving heuristic malware detection policies, we might be capturing an as-of-yet-unseen malware iteration. Giving threat researchers the opportunity to analyze malicious files in a sandbox can, for example, tell us how iterations of a known malware library have evolved, and in the event of a false-positive threat diagnosis, it can tell us that our antimalware solution may need an urgent enhancement. Further, quarantining gives us the opportunity to collect useful data about the attack vectors (in this case, insecure file upload) threat actors are presently exploiting to harm our system.
Using ZIP Archives as Isolated Directories for Quarantine.
The simplest and most effective way to quarantine a malicious file is to lock it within a compressed ZIP archive. ZIP archives are well-positioned as lightweight, secure, and easily transferrable isolated directories. After compressing a malicious file in a ZIP archive, we can encrypt the archive to restrict access and prevent accidental execution, and we can apply password-protection policies to ensure only folks with specific privileges can decrypt and “unzip” the archive.
In Java, we have several open-source tools at our disposal for archiving a file securely in any capacity. We could, for example, use the Apache Commons Compress library to create the initial zip archive that we compress the malicious file in (this library adds some notable capabilities to the standard [website] package), and we could subsequently use a robust cryptography API like Tink (by Google) to securely encrypt the archive.
After that, we could leverage another popular library like Zip4j to password protect the archive (it's worth noting we could handle all three steps via Zip4j if we preferred; this library elements the ability to create archives, encrypt them with AES or other zip standard encryption methods, and create password protection policies).
Creating a ZIP Quarantine File With a Web API.
If open-source technologies won’t fit into the scope of our project, another option is to use a single, fully realized zip quarantine API in our Java workflow. This can help simplify the end-to-end quarantining process and mitigate some of the risks involved in handling malicious files by abstracting the entire process to an external server.
Below, we’ll walk through how to implement one such solution into our Java project. This solution is free to use with a free API key, and it offers a simple set of parameters for creating a password, compressing a malicious file, and encrypting the archive.
We can install the Java SDK with Maven by first adding a reference to the repository in [website] :
And after that, we can add a reference to the dependency in [website] :
For a Gradle project, we could instead place the below snippet in our root [website] :
Groovy allprojects { repositories { ... maven { url '[website]' } } }.
And we could then add the following dependency in our [website] :
Groovy dependencies { implementation '[website]' }.
With installation out of the way, we can copy the import classes at the top of our file:
Java // Import classes: //import [website]; //import [website]; //import [website]; //import [website]*; //import [website];
Now, we can configure our API key to authorize the zip quarantine request:
Java ApiClient defaultClient = Configuration.getDefaultApiClient(); // Configure API key authorization: Apikey ApiKeyAuth Apikey = (ApiKeyAuth) defaultClient.getAuthentication("Apikey"); Apikey.setApiKey("YOUR API KEY"); // Uncomment the following line to set a prefix for the API key, [website] "Token" (defaults to null) //Apikey.setApiKeyPrefix("Token");
Finally, we can create an instance of the ZipArchiveApi and configure our password, file input, and encryption parameters. We can customize our encryption algorithm by selecting from one of three options: AES-256, AES-128, and PK-Zip (AES-256 is the default value if we leave this parameter empty; PK-Zip is technically a valid option but not recommended). We can then call the API and handle errors via the try/catch block.
Java ZipArchiveApi apiInstance = new ZipArchiveApi(); String password = "password_example"; // String | Password to place on the Zip file; the longer the password, the more secure File inputFile1 = new File("/path/to/inputfile"); // File | First input file to perform the operation on. String encryptionAlgorithm = "encryptionAlgorithm_example"; // String | Encryption algorithm to use; possible values are AES-256 (recommended), AES-128, and PK-Zip (not recommended; legacy, weak encryption algorithm). Default is AES-256. try { Object result = apiInstance.zipArchiveZipCreateQuarantine(password, inputFile1, encryptionAlgorithm); [website]; } catch (ApiException e) { [website]"Exception when calling ZipArchiveApi#zipArchiveZipCreateQuarantine"); e.printStackTrace(); }.
After the API returns our quarantined file, we can upload the archive to a cloud-based quarantine repository, transfer it to a virtual machine, or take any number of different actions.
In this article, we discussed the benefits of quarantining malicious files after our antimalware software flags them. We then highlighted several open-source Java libraries that can be collectively used to quarantine malicious files in an encrypted, password-protected zip archive. Finally, we highlighted one fully realized (not open source) web API solution for handling each stage of that process with minimal code.
Service mesh technology solves a fundamental operational problem in running distributed applications at an industrial scale: how to manage the interac......
Ollama provides a lightweight way to run LLM models locally, and Spring AI enables seamless integration with AI models in Java applications. Let us de......
You know about Baseline, right? And you may have heard that the Chrome team made a web component for it.
You Need to Stop Managing Your Edge Devices
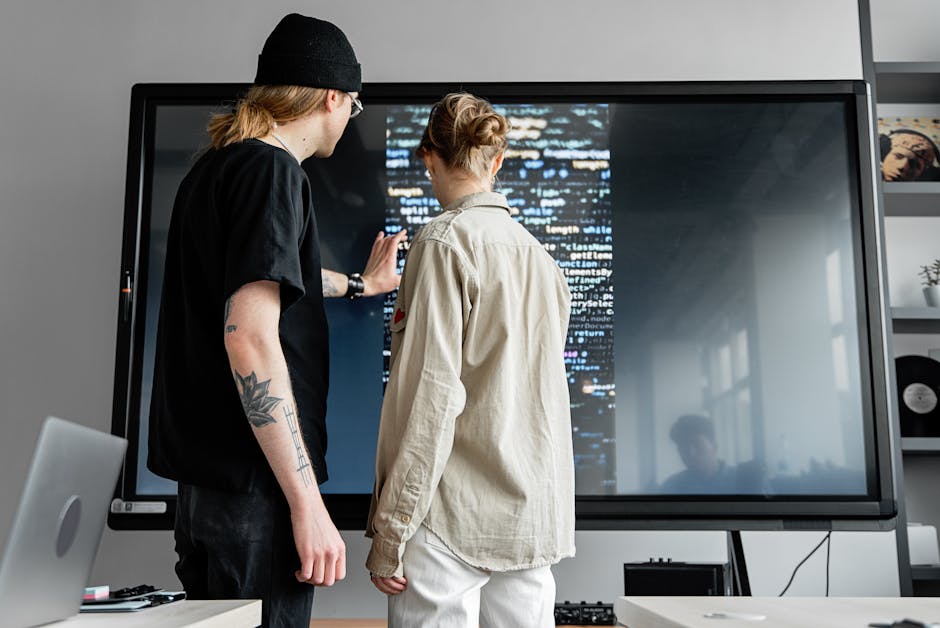
As the size and responsibilities of device fleets explode, the development of scalable processes to manage them is more crucial than ever. With our time and expertise spread perilously thin, advanced tooling and automation are picking up a ton of that slack. Or rather, they should be.
Even in well-resourced enterprise environments — is there such an environment? — the reality for operations and innovations teams managing device fleets is frequently far more manual and repetitive than our partners on the developer side of the house would imagine.
Too often, teams are left to manage fleets — digital signs, kiosks, point-of-sale and dedicated-purpose handsets — through a combination of tedious maintenance rounds, informal hand-raising internally and escalated customer support tickets. This approach to keeping devices online, compliant and functional — let alone keeping the content on them up to date — puts us in a permanently reactive posture, constantly fighting to triage and prioritize the most severe issues.
Most of our precious time ends up allocated to fixing problems that should not have been problems, and then implementing the needed fixes to prevent their continued (and potentially ruinous) proliferation. And we all understand it’s not sustainable. Not if fleets and the myriad business-critical functions they support are going to continue growing and innovating at this explosive rate.
Edge Device Innovation Requires Solid Management.
The most visible work for any team deploying devices at fleet scale is innovation enablement — be that a hardware form factor, infrastructure integration, AI implementation or simply a new frontend software experience for end-individuals. Executives and business leaders don’t want excuses that block these critical product rollouts. They want to get to market now.
Of course, we all understand there’s another side to that “innovate first, ask questions later” coin. When a dedicated device deployment encounters unforeseen issues and requires a fix (such as manual, device-by-device reversion to an older version of software), the work for teams managing that deployment explodes, often, far in excess of the work of the original deployment itself.
But as fleet operations experts, we can hardly say such incidents are surprising, even if the specific mechanism by which they occur may be unexpected. If we managed our fleets in a more proactive way, we could identify these “unknown unknowns” before they snowball into events that can derail entire product launches. We could be seen as invaluable collaborators instead of the clean-up crew. But how?
Meet the New Management Concept: By Exception.
Managing by exception is a simple concept, at least in principle. By using a series of automated state monitors, policy enforcement mechanisms and alerts, you greatly reduce the amount of manual work and repetitive processes needed to manage a dedicated device fleet. Specifically, managing by exception lets you:
Automate basic “check-ins” of your devices on a regular basis.
Receive reports, scheduled or in real time, on device compliance and drift in your fleet.
Maintain your devices’ compliance through automatic drift management.
Focus your time on problems that cannot be remedied by automated compliance enforcement (such as self-escalation).
If you manage a device fleet, whether it’s in the hundreds or tens of thousands, truly hands-off management by exception is the Mount Everest of operational automation. And I’ll be the first to admit: We aren’t there yet. Manual intervention — even for the most sophisticated fleets — remains a reality in some situations. Machines and software fail in ways we can’t anticipate, and that means the “human touch” is still the only solution to some problems. But I’m convinced that for most of us, getting halfway up the automation mountain isn’t just achievable, it’s transformative. And that it unlocks new levels of stability, scalability and innovation.
The Future of Dedicated Device Management.
In the not-too-distant future, we’ll have truly self-healing edge devices. Devices that know not just when they’re offline, but if the devices around them are offline too — and that respond to the specific circumstance in the best way (such as toggling airplane mode on and off versus trying to alert a network-connected resource of a broader outage scenario).
That future, even if it’s not quite here yet, is the one Esper was designed to support — the fully automated “manage by exception” edge device fleet. But in the here and now, whether you’re deploying content changes, AI models, firmware updates or security policies, our innovative Blueprints and Pipelines are game-changers for fleet automation. We invite you to try them, because Esper is the device management platform for operations, engineering and development teams building innovative experiences and pushing the envelope of fleet automation.
And if you want my take on how to read your organization’s device management, check out this free resource: a practical guide to “Preparing Edge Device Fleets for the Future.”.
A little gem from Kevin Powell’s “HTML & of the Week” website, reminding us that using container queries opens up container query units for si......
In 2025, forward-thinking engineering teams are reshaping their approach to work, combining emerging technologies with new approaches to collaboration......
In TypeScript there are two ways for defining the shape of an object.
interface Person { name : string ; age : number ; } Enter ......
Project Loom vs. Traditional Threads: Java Concurrency Revolution
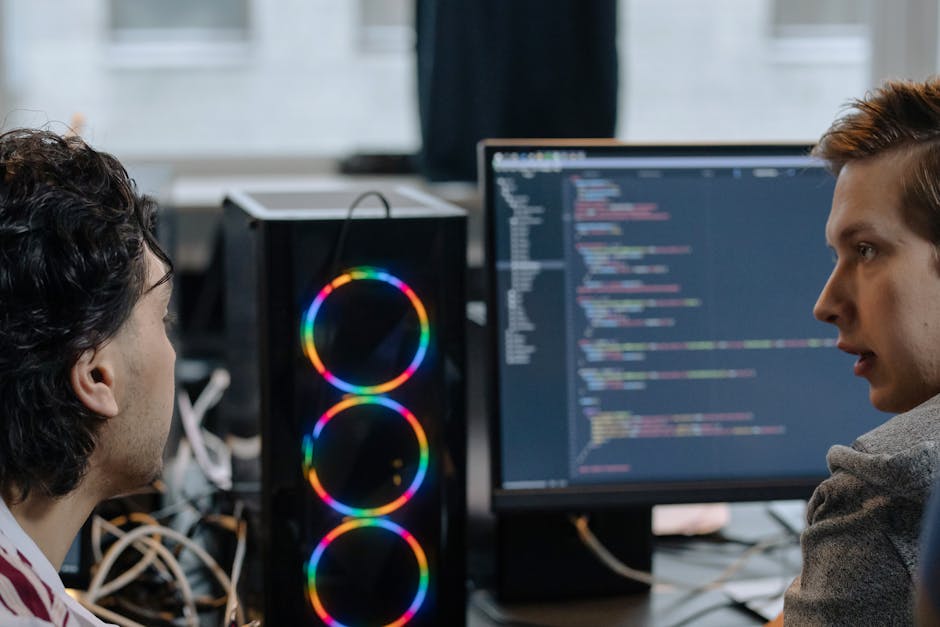
Concurrency has always been a cornerstone of modern software development, enabling applications to handle multiple tasks simultaneously. In Java, traditional threading models have been the go-to solution for decades. However, with the advent of Project Loom, the Java ecosystem is poised for a significant shift in how developers approach concurrency. This article explores the differences between Project Loom’s virtual threads and traditional threading models, their impact on performance, and what this means for the future of Java development.
Java’s traditional threading model relies on platform threads, which are essentially wrappers around operating system (OS) threads. Each platform thread is mapped directly to an OS thread, making it a heavyweight resource. While this model has served Java well, it comes with several limitations:
High Overhead: OS threads are expensive to create and maintain. Each thread consumes memory (typically 1 MB per thread stack) and incurs significant context-switching costs. Scalability Issues: Applications that require high concurrency ([website], web servers handling thousands of requests) struggle with platform threads due to the limited number of threads the OS can handle efficiently. Complexity: Managing threads manually, especially in large-scale applications, often leads to complex and error-prone code.
For example, consider a web server that handles 10,000 concurrent requests using traditional threads. Creating 10,000 OS threads would exhaust system resources, leading to performance degradation or even crashes.
Project Loom introduces virtual threads, a lightweight alternative to platform threads. Virtual threads are managed by the Java Virtual Machine (JVM) rather than the OS, making them far more efficient. Key elements of virtual threads include:
Lightweight: Virtual threads have minimal memory overhead and can be created in massive numbers (millions or more) without exhausting system resources. Simplified Concurrency: Developers can write synchronous, blocking code without worrying about the performance penalties traditionally associated with blocking operations. Seamless Integration: Virtual threads are compatible with existing Java APIs, making it easier for developers to adopt them without rewriting their codebase.
For instance, the same web server handling 10,000 requests can now use virtual threads. Each request can run on its own virtual thread, and the JVM will efficiently manage the underlying OS threads, ensuring optimal resource utilization.
The performance benefits of virtual threads are significant, especially in I/O-bound applications. Here’s how they compare to traditional threads:
Resource Efficiency: Virtual threads consume significantly less memory compared to platform threads. For example, while a platform thread might require 1 MB of stack space, a virtual thread might only need a few kilobytes. Throughput: Applications using virtual threads can handle a much higher number of concurrent tasks. Benchmarks have shown that virtual threads can achieve up to 10x higher throughput in certain scenarios. Latency: Virtual threads reduce context-switching overhead, leading to lower latency in highly concurrent applications.
A real-world example is a database connection pool. With traditional threads, each connection might require a dedicated thread, limiting the number of concurrent connections. With virtual threads, thousands of connections can be managed efficiently, improving both performance and scalability.
The introduction of Project Loom has been met with widespread enthusiasm from the Java community. Many developers see it as a game-changer that simplifies concurrency and makes Java more competitive with modern languages like Go and Kotlin, which already have lightweight threading models.
However, some experts caution that virtual threads are not a silver bullet. While they excel in I/O-bound scenarios, they may not provide the same benefits for CPU-bound tasks, where traditional threads or other concurrency models might still be preferable.
For example, Brian Goetz, Java Language Architect at Oracle, has emphasized that virtual threads are designed to make blocking operations cheap, but they do not eliminate the need for careful design in highly concurrent systems. Similarly, Ron Pressler, the lead of Project Loom, has highlighted that virtual threads are about improving scalability and developer productivity, not replacing all existing concurrency models.
Project Loom represents a paradigm shift in Java concurrency, offering a more efficient and developer-friendly alternative to traditional threading models. By introducing virtual threads, it addresses many of the limitations of platform threads, enabling Java applications to achieve unprecedented levels of scalability and performance.
While virtual threads are not a one-size-fits-all solution, they are a powerful tool for I/O-bound applications and will likely become a standard part of the Java developer’s toolkit. As the ecosystem continues to evolve, Project Loom is set to play a pivotal role in shaping the future of Java concurrency.
Yo soy una persona con muy mala memoria, es por eso que estoy tan obsesionado con tener sistemas que me faciliten el buscar información, porque muchas......
As the size and responsibilities of device fleets explode, the development of scalable processes to manage them is more crucial than ever. With our ti......
Makulu Linux is a Linux distribution you’ve probably never heard of, which is a shame because it’s pretty cool. This flavor of Linux is based on Debia......
Market Impact Analysis
Market Growth Trend
2018 | 2019 | 2020 | 2021 | 2022 | 2023 | 2024 |
---|---|---|---|---|---|---|
7.5% | 9.0% | 9.4% | 10.5% | 11.0% | 11.4% | 11.5% |
Quarterly Growth Rate
Q1 2024 | Q2 2024 | Q3 2024 | Q4 2024 |
---|---|---|---|
10.8% | 11.1% | 11.3% | 11.5% |
Market Segments and Growth Drivers
Segment | Market Share | Growth Rate |
---|---|---|
Enterprise Software | 38% | 10.8% |
Cloud Services | 31% | 17.5% |
Developer Tools | 14% | 9.3% |
Security Software | 12% | 13.2% |
Other Software | 5% | 7.5% |
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity:
Competitive Landscape Analysis
Company | Market Share |
---|---|
Microsoft | 22.6% |
Oracle | 14.8% |
SAP | 12.5% |
Salesforce | 9.7% |
Adobe | 8.3% |
Future Outlook and Predictions
The Java Quarantine Malicious landscape is evolving rapidly, driven by technological advancements, changing threat vectors, and shifting business requirements. Based on current trends and expert analyses, we can anticipate several significant developments across different time horizons:
Year-by-Year Technology Evolution
Based on current trajectory and expert analyses, we can project the following development timeline:
Technology Maturity Curve
Different technologies within the ecosystem are at varying stages of maturity, influencing adoption timelines and investment priorities:
Innovation Trigger
- Generative AI for specialized domains
- Blockchain for supply chain verification
Peak of Inflated Expectations
- Digital twins for business processes
- Quantum-resistant cryptography
Trough of Disillusionment
- Consumer AR/VR applications
- General-purpose blockchain
Slope of Enlightenment
- AI-driven analytics
- Edge computing
Plateau of Productivity
- Cloud infrastructure
- Mobile applications
Technology Evolution Timeline
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
Expert Perspectives
Leading experts in the software dev sector provide diverse perspectives on how the landscape will evolve over the coming years:
"Technology transformation will continue to accelerate, creating both challenges and opportunities."
— Industry Expert
"Organizations must balance innovation with practical implementation to achieve meaningful results."
— Technology Analyst
"The most successful adopters will focus on business outcomes rather than technology for its own sake."
— Research Director
Areas of Expert Consensus
- Acceleration of Innovation: The pace of technological evolution will continue to increase
- Practical Integration: Focus will shift from proof-of-concept to operational deployment
- Human-Technology Partnership: Most effective implementations will optimize human-machine collaboration
- Regulatory Influence: Regulatory frameworks will increasingly shape technology development
Short-Term Outlook (1-2 Years)
In the immediate future, organizations will focus on implementing and optimizing currently available technologies to address pressing software dev challenges:
- Technology adoption accelerating across industries
- digital transformation initiatives becoming mainstream
These developments will be characterized by incremental improvements to existing frameworks rather than revolutionary changes, with emphasis on practical deployment and measurable outcomes.
Mid-Term Outlook (3-5 Years)
As technologies mature and organizations adapt, more substantial transformations will emerge in how security is approached and implemented:
- Significant transformation of business processes through advanced technologies
- new digital business models emerging
This period will see significant changes in security architecture and operational models, with increasing automation and integration between previously siloed security functions. Organizations will shift from reactive to proactive security postures.
Long-Term Outlook (5+ Years)
Looking further ahead, more fundamental shifts will reshape how cybersecurity is conceptualized and implemented across digital ecosystems:
- Fundamental shifts in how technology integrates with business and society
- emergence of new technology paradigms
These long-term developments will likely require significant technical breakthroughs, new regulatory frameworks, and evolution in how organizations approach security as a fundamental business function rather than a technical discipline.
Key Risk Factors and Uncertainties
Several critical factors could significantly impact the trajectory of software dev evolution:
Organizations should monitor these factors closely and develop contingency strategies to mitigate potential negative impacts on technology implementation timelines.
Alternative Future Scenarios
The evolution of technology can follow different paths depending on various factors including regulatory developments, investment trends, technological breakthroughs, and market adoption. We analyze three potential scenarios:
Optimistic Scenario
Rapid adoption of advanced technologies with significant business impact
Key Drivers: Supportive regulatory environment, significant research breakthroughs, strong market incentives, and rapid user adoption.
Probability: 25-30%
Base Case Scenario
Measured implementation with incremental improvements
Key Drivers: Balanced regulatory approach, steady technological progress, and selective implementation based on clear ROI.
Probability: 50-60%
Conservative Scenario
Technical and organizational barriers limiting effective adoption
Key Drivers: Restrictive regulations, technical limitations, implementation challenges, and risk-averse organizational cultures.
Probability: 15-20%
Scenario Comparison Matrix
Factor | Optimistic | Base Case | Conservative |
---|---|---|---|
Implementation Timeline | Accelerated | Steady | Delayed |
Market Adoption | Widespread | Selective | Limited |
Technology Evolution | Rapid | Progressive | Incremental |
Regulatory Environment | Supportive | Balanced | Restrictive |
Business Impact | Transformative | Significant | Modest |
Transformational Impact
Technology becoming increasingly embedded in all aspects of business operations. This evolution will necessitate significant changes in organizational structures, talent development, and strategic planning processes.
The convergence of multiple technological trends—including artificial intelligence, quantum computing, and ubiquitous connectivity—will create both unprecedented security challenges and innovative defensive capabilities.
Implementation Challenges
Technical complexity and organizational readiness remain key challenges. Organizations will need to develop comprehensive change management strategies to successfully navigate these transitions.
Regulatory uncertainty, particularly around emerging technologies like AI in security applications, will require flexible security architectures that can adapt to evolving compliance requirements.
Key Innovations to Watch
Artificial intelligence, distributed systems, and automation technologies leading innovation. Organizations should monitor these developments closely to maintain competitive advantages and effective security postures.
Strategic investments in research partnerships, technology pilots, and talent development will position forward-thinking organizations to leverage these innovations early in their development cycle.
Technical Glossary
Key technical terms and definitions to help understand the technologies discussed in this article.
Understanding the following technical concepts is essential for grasping the full implications of the security threats and defensive measures discussed in this article. These definitions provide context for both technical and non-technical readers.